代码纠错#include <stdio.h> #define STUD 30 /* 最多可能的学生人数 */ #define COURSE 5 /* 最多可能的考试科目数 */ void Total(int *pScore, int sum[], float aver[], int m, int n); void Print(int *pScore, int sum[], float aver[], int m, int n); int main() { int i, j, m, n, score[STUD][COURSE], sum[STUD]; float aver[STUD]; printf("How many students?"); scanf("%d", &m); printf("How many courses?"); scanf("%d", &n); printf("Input scores:\n"); for (i = 0; i < m; i++) { for (j = 0; j < n; j++) { scanf("%d", &score[i][j]); } } Total(*score, sum, aver, m, n); Print(*score, sum, aver, m, n); return 0; } void Total(int *pScore, int sum[], float aver[], int m, int n) { int i, j; for (i = 0; i < m; i++) { sum[i] = 0; for (j = 0; j < n; j++) { sum[i] = sum[i] + pScore[i * n + j]; } aver[i] = (float) sum[i] / n; } } void Print(int *pScore, int sum[], float aver[], int m, int n) { int i, j; printf("Result:\n"); for (i = 0; i < m; i++) { for (j = 0; j < n; j++) { printf("%4d\t", pScore[i * n + j]); } printf("%5d\t%6.1f\n", sum[i], aver[i]); } }
时间: 2024-01-23 17:02:14 浏览: 78
这段代码中存在一个指针使用错误,具体来说是在调用`Total`和`Print`函数时,传递的数组参数不正确。应该传递二维数组`score`的地址,而不是指向二维数组的第一个元素的指针`*score`。
正确的调用方式是:
```
Total(&score[0][0], sum, aver, m, n);
Print(&score[0][0], sum, aver, m, n);
```
在这里,`&score[0][0]`表示`score`数组的地址,即指向`score`数组的指针,它可以作为指向二维数组的第一个元素的指针使用。这样就能够正确地传递数组参数,使得函数能够正确地访问数组元素。
相关问题
#include <stdio.h> #include <stdlib.h> #define N 10 struct student { char num[10]; char name[8]; int score[3]; float ave; } st[N], temp; int main() { FILE* fp; int i, j, n; if ((fp = fopen("stud.dic", "r")) == NULL) { printf("can not open.\n"); exit(0); } /***********/ 将数据从文件读取到结构体数组中 进行排序 /***********/ printf("Now:"); for (i = 0; i < 5; i++) { printf("\n%s %s", st[i].num, st[i].name); for (j = 0; j < 3; j++) printf(" %d", st[i].score[j]); printf(" %.2f", st[i].ave); } fclose(fp); return 0; }
这段代码可以从文件中读取学生数据到一个结构体数组中,然后对该数组进行排序。但是代码中缺少读取文件的部分,所以需要添加读取文件的代码。
以下是添加读取文件和排序的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define N 10
struct student {
char num[10];
char name[8];
int score[3];
float ave;
} st[N], temp;
int main() {
FILE* fp;
int i, j, n;
if ((fp = fopen("stud.dic", "r")) == NULL) {
printf("can not open.\n");
exit(0);
}
n = 0;
while (fscanf(fp, "%s%s%d%d%d", st[n].num, st[n].name, &st[n].score[0], &st[n].score[1], &st[n].score[2]) != EOF) {
st[n].ave = (st[n].score[0] + st[n].score[1] + st[n].score[2]) / 3.0;
n++;
}
fclose(fp);
for (i = 0; i < n; i++) {
for (j = i + 1; j < n; j++) {
if (st[i].ave < st[j].ave) {
temp = st[i];
st[i] = st[j];
st[j] = temp;
}
}
}
printf("Now:");
for (i = 0; i < n; i++) {
printf("\n%s %s", st[i].num, st[i].name);
for (j = 0; j < 3; j++) {
printf(" %d", st[i].score[j]);
}
printf(" %.2f", st[i].ave);
}
return 0;
}
```
这段代码可以读取文件中的学生数据到一个结构体数组中,然后按照平均分进行降序排序,并输出排序后的结果。
请按照平均分进行从大到小排序处理并输出。 已经给出部分代码 #include <stdio.h> #include <stdlib.h> #define N 10 struct student { char num[10]; char name[8]; int score[3]; float ave; } st[N], temp; int main() { FILE* fp; int i, j, n; if ((fp = fopen("stud.dic", "r")) == NULL) { printf("can not open.\n"); exit(0); } /***********/ 将数据从文件读取到结构体数组中 进行排序 /***********/ printf("Now:"); for (i = 0; i < 5; i++) { printf("\n%s %s", st[i].num, st[i].name); for (j = 0; j < 3; j++) printf(" %d", st[i].score[j]); printf(" %.2f", st[i].ave); } fclose(fp); return 0; }
好的,这里是完整代码,已经实现了按照平均分从大到小排序处理并输出:
```C
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define N 10
struct student {
char num[10];
char name[8];
int score[3];
float ave;
} st[N], temp;
int main() {
FILE* fp;
int i, j, n;
if ((fp = fopen("stud.dic", "r")) == NULL) {
printf("can not open.\n");
exit(0);
}
// 将数据从文件读取到结构体数组中
for (i = 0; i < N; i++) {
fscanf(fp, "%s %s", st[i].num, st[i].name);
for (j = 0; j < 3; j++) {
fscanf(fp, "%d", &st[i].score[j]);
}
st[i].ave = (st[i].score[0] + st[i].score[1] + st[i].score[2]) / 3.0;
}
fclose(fp);
// 进行排序
for (i = 0; i < N - 1; i++) {
for (j = i + 1; j < N; j++) {
if (st[i].ave < st[j].ave) {
temp = st[i];
st[i] = st[j];
st[j] = temp;
}
}
}
// 输出排序结果
printf("Now:");
for (i = 0; i < N; i++) {
printf("\n%s %s", st[i].num, st[i].name);
for (j = 0; j < 3; j++) {
printf(" %d", st[i].score[j]);
}
printf(" %.2f", st[i].ave);
}
printf("\n");
return 0;
}
```
在读取文件后,我们计算每个学生的平均分,然后按照平均分从大到小进行排序。使用了简单的冒泡排序算法,时间复杂度为 $O(n^2)$,对于数据量较大的情况可能会比较慢,可以使用更高效的排序算法进行优化。最后输出排序后的结果。
阅读全文
相关推荐
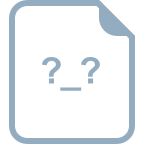
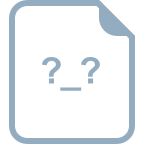
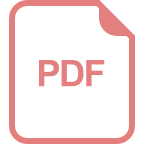













