如何使用html实现搜索栏以列表形式的提示词下拉
时间: 2024-02-06 19:10:23 浏览: 139
可以通过以下步骤实现:
1. 创建一个包含搜索栏的HTML表单。
2. 在表单中添加一个文本框,使用JavaScript和CSS创建下拉提示列表。
3. 使用JavaScript编写一个函数,该函数将获取文本框中的值并在下拉提示列表中搜索匹配项。
4. 将匹配项显示在下拉提示列表中。
5. 当用户选择下拉提示列表中的一个项目时,将其值插入到文本框中。
6. 可以使用CSS对下拉提示列表进行样式设置,例如字体大小、背景颜色和边框。
以下是一个示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>Search Bar with Dropdown Suggestions</title>
<style>
#search-box {
position: relative;
display: inline-block;
}
#search-box input[type="text"] {
width: 100%;
padding: 10px;
border: 1px solid #CCCCCC;
border-radius: 5px;
}
#search-box ul {
position: absolute;
top: 100%;
left: 0;
width: 100%;
border: 1px solid #CCCCCC;
border-top: none;
border-bottom-left-radius: 5px;
border-bottom-right-radius: 5px;
padding: 0;
margin: 0;
list-style: none;
display: none;
background-color: #FFFFFF;
z-index: 999;
}
#search-box ul li {
padding: 10px;
cursor: pointer;
}
#search-box ul li:hover {
background-color: #F5F5F5;
}
</style>
</head>
<body>
<form>
<div id="search-box">
<input type="text" placeholder="Search...">
<ul></ul>
</div>
</form>
<script>
var searchBox = document.getElementById("search-box");
var inputBox = searchBox.getElementsByTagName("input")[0];
var suggestionBox = searchBox.getElementsByTagName("ul")[0];
inputBox.addEventListener("keyup", function() {
var inputValue = inputBox.value;
suggestionBox.innerHTML = "";
if (inputValue.length > 0) {
suggestionBox.style.display = "block";
// Replace with your own search function
var suggestions = ["apple", "banana", "cherry", "date", "elderberry"];
for (var i = 0; i < suggestions.length; i++) {
if (suggestions[i].toLowerCase().indexOf(inputValue.toLowerCase()) > -1) {
var suggestionItem = document.createElement("li");
suggestionItem.appendChild(document.createTextNode(suggestions[i]));
suggestionBox.appendChild(suggestionItem);
}
}
} else {
suggestionBox.style.display = "none";
}
});
suggestionBox.addEventListener("click", function(e) {
inputBox.value = e.target.innerText;
suggestionBox.style.display = "none";
});
</script>
</body>
</html>
```
在这个示例中,我们使用CSS设置了搜索栏和下拉提示列表的样式。在JavaScript代码中,我们添加了一个键盘事件监听器来处理用户输入,并在下拉提示列表中显示匹配项。当用户点击下拉提示列表中的一个项目时,我们将其文本值插入到搜索栏文本框中。最后,我们使用CSS将下拉提示列表隐藏起来,直到需要显示匹配项时才显示出来。
阅读全文
相关推荐
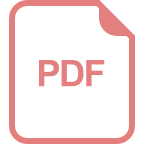
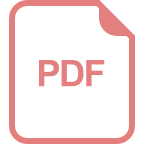
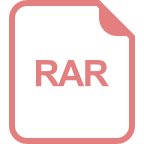















