从数据库中读取附带图片名称的数据,controller层在html中动态生成布局的案例
时间: 2024-06-11 17:10:38 浏览: 109
假设我们有一个数据库表格,里面存储了商品的信息,包括商品的名称、价格和对应的图片名称。我们需要从数据库中读取这些数据,然后在前端页面中动态生成布局,展示商品的信息和对应的图片。
首先,我们需要在controller层中定义一个API接口,用于从数据库中读取商品信息。假设我们使用Spring Boot框架,这个API接口的代码可能如下所示:
```
@RestController
@RequestMapping("/api/products")
public class ProductController {
@Autowired
private ProductService productService;
@GetMapping("")
public List<Product> getAllProducts() {
return productService.getAllProducts();
}
}
```
这里我们使用了@Autowired注解来注入ProductService对象,ProductService是一个服务层组件,用于调用数据访问层的代码,从数据库中读取商品信息。
接下来,我们需要在html页面中动态生成布局,展示商品信息和对应的图片。假设我们使用Bootstrap框架来设计页面,这个页面的代码可能如下所示:
```
<!DOCTYPE html>
<html>
<head>
<title>Product List</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.16.0/umd/popper.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js"></script>
</head>
<body>
<div class="container">
<h2>Product List</h2>
<div class="row">
<div class="col-md-4" v-for="product in products">
<div class="card mb-4 shadow-sm">
<img class="card-img-top" :src="'/images/' + product.imageName" alt="Product image">
<div class="card-body">
<h5 class="card-title">{{ product.name }}</h5>
<p class="card-text">{{ product.price }}</p>
<div class="d-flex justify-content-between align-items-center">
<div class="btn-group">
<button type="button" class="btn btn-sm btn-outline-secondary">View</button>
<button type="button" class="btn btn-sm btn-outline-secondary">Edit</button>
</div>
<small class="text-muted">9 mins</small>
</div>
</div>
</div>
</div>
</div>
</div>
<script>
var app = new Vue({
el: '#app',
data: {
products: []
},
mounted: function () {
this.getProducts();
},
methods: {
getProducts: function () {
axios.get('/api/products').then(response => {
this.products = response.data;
}).catch(error => {
console.log(error);
});
}
}
});
</script>
</body>
</html>
```
这里我们使用了Vue.js框架来实现动态绑定数据,使用axios库来发送GET请求,从后端API接口中获取商品信息。在HTML代码中,我们使用了Bootstrap的网格系统来实现响应式布局,将每个商品展示在一个卡片上,包括商品名称、价格和对应的图片。
最后,我们需要在Spring Boot的配置文件中添加静态资源路径的配置,让后端能够访问到存储在本地的图片文件。这个配置的代码可能如下所示:
```
spring.resources.static-locations=classpath:/static/,file:/path/to/images/
```
这里我们将静态资源的路径设置为classpath:/static/和file:/path/to/images/,前者是用于访问前端页面中使用的静态资源,后者是用于访问存储在本地的图片文件。
以上就是一个从数据库中读取附带图片名称的数据,controller层在html中动态生成布局的案例。
阅读全文
相关推荐
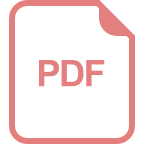
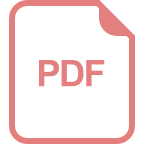
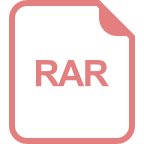
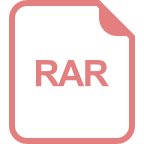
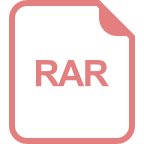
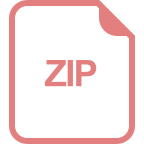
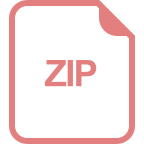
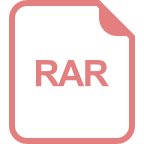
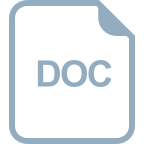
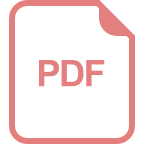
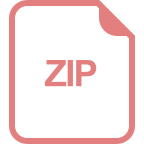
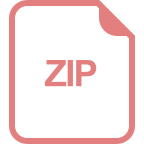
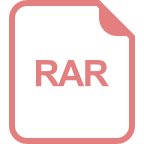