n_steps = 10 # 窗口大小为 10 n_length = 1 # 输出序列长度为 1 n_features = 5 # 特征数为 5 # Create a model def create_LSTM_model(X_train,n_steps,n_length, n_features): # instantiate the model model = Sequential() model.add(Input(shape=(X_train.shape[1], X_train.shape[2]))) X_train = X_train.reshape((X_train.shape[0], n_steps, 1, n_length, n_features)) model.add(ConvLSTM2D(filters=64, kernel_size=(1,3), activation='relu', input_shape=(n_steps, 1, n_length, n_features))) model.add(Flatten()) # cnn1d Layers # 添加lstm层 model.add(LSTM(64, activation = 'relu', return_sequences=True)) model.add(Dropout(0.5)) #添加注意力层 model.add(LSTM(64, activation = 'relu', return_sequences=False)) # 添加dropout model.add(Dropout(0.5)) model.add(Dense(128)) # 输出层 model.add(Dense(1, name='Output')) # 编译模型 model.compile(optimizer='adam', loss='mse', metrics=['mae']) return model # lstm network model = create_LSTM_model(X_train,n_steps,n_length, n_features) # summary print(model.summary())修改该代码,解决ValueError: Input 0 of layer "conv_lstm2d_11" is incompatible with the layer: expected ndim=5, found ndim=3. Full shape received: (None, 10, 5)问题
时间: 2023-12-09 14:04:53 浏览: 37
将以下代码:
```
X_train = X_train.reshape((X_train.shape[0], n_steps, 1, n_length, n_features))
```
修改为:
```
X_train = X_train.reshape((X_train.shape[0], n_steps, n_features))
```
这样就可以解决该错误。同时,注意修改后输入层的shape也需要相应地修改为:
```
model.add(Input(shape=(n_steps, n_features)))
```
相关问题
n_steps_in = 10 和n_steps_out = 180 的作用
这两个参数通常用于序列预测模型中,其中n_steps_in是指输入序列的时间步数,而n_steps_out是指输出序列的时间步数。具体来说,n_steps_in表示模型需要观察多少个时间步的输入数据,才能预测下一个时间步的输出。n_steps_out则表示模型需要预测未来多少个时间步的输出。
例如,假设你要构建一个股票价格预测模型,每天的股票价格作为一个数据点,你想预测未来30天的股票价格走势。那么n_steps_in就应该是过去10天的股票价格数据,而n_steps_out应该是未来30天的股票价格数据。
这些参数的设置对模型的性能和预测能力有很大影响,需要根据具体问题和数据来进行调整。
给出定义n_steps, 1, n_length, n_features的代码
以下是一种可能的定义:
```python
n_steps = 10 # 时间步数,表示序列中有多少个时间步
n_length = 20 # 每个时间步中的序列长度,表示每个时间步的输入或输出是一个长度为20的向量
n_features = 30 # 每个向量的特征数,表示每个向量有30个特征
# 假设有一个形状为 (batch_size, n_steps, n_length, n_features) 的输入张量 x
# 可以使用以下代码获取张量的各个维度信息
batch_size = x.shape[0]
n_steps = x.shape[1]
n_length = x.shape[2]
n_features = x.shape[3]
```
相关推荐
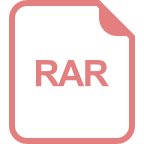
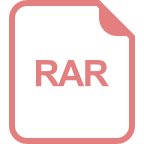













