图片中有一长条纹状的焊接轨迹,如何利用OpenCVsharp4对焊缝进行识别,并大致算出长条纹相对于垂直方向的偏角
时间: 2024-10-24 22:09:37 浏览: 18
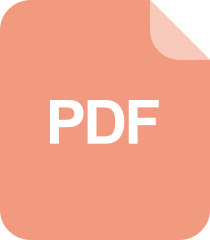
基于海森矩阵与区域增长的激光条纹中心提取
为了在OpenCVSharp 4中识别图片中的焊缝轨迹并计算其偏角,你可以按照以下步骤操作:
1. **预处理**:
- 对图片进行灰度化处理,去除颜色干扰,提高对比度。
```csharp
Mat grayImage = new Mat();
Cv2.CvtColor(image, grayImage, ColorConversionCodes.BGR2GRAY);
```
2. **边缘检测**:
- 使用Canny边检测算法找出焊缝轨迹的边缘。
```csharp
Mat edges;
Canny(grayImage, edges, lowThreshold, highThreshold);
```
3. **腐蚀和膨胀**:
- 有时焊缝可能会因为噪声而断裂,所以可以先腐蚀后膨胀来连接断裂的部分。
```csharp
Mat kernel = GetStructuringElement(ElementShape.MORPH_RECT, new Size(3, 3));
Mat tempEdges;
Cv2.Dilate(edges, tempEdges, kernel);
Cv2.Erode(tempEdges, edges, kernel);
```
4. **找到焊缝轨迹**:
- 使用`findContours`函数寻找连续的线段,然后选择最宽的一条作为焊缝。
```csharp
List<MatOfPoint> contours = new List<MatOfPoint>();
Cv2.findContours(edges, contours, RetrType.List, ChainApproxMethod.ChainApprox_SIMPLE);
int widestContourIndex = FindWidestContour(contours);
var contour = contours[widestContourIndex];
```
5. **计算偏角**:
- 使用`fitLine()`函数拟合焊缝轨迹,并计算与垂直方向的夹角。
```csharp
Point2f[] points = new Point2f[contour.Count];
for (int i = 0; i < contour.Size(); i++)
points[i] = contour.Get(i)[0];
LineSegment2D line = new LineSegment2D(points);
double angle = Math.Atan2(line.y2 - line.y1, line.x2 - line.x1) * 180 / Math.PI;
double verticalAngle = Math.Abs(angle - 90);
```
注意:这里假设垂直方向的偏角是指从垂直线向上偏离的角度。
6. **角度归一化**:
- 如果角度不是正数(即向下偏离),需要调整角度范围至0到180度之间。
```csharp
if (verticalAngle < 0)
verticalAngle += 180;
```
阅读全文
相关推荐
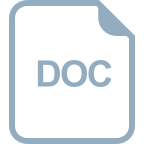
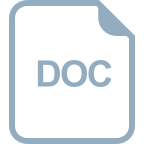
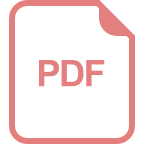
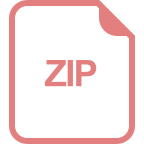
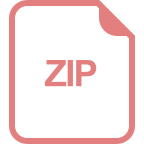
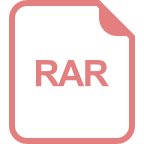
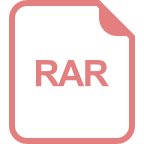
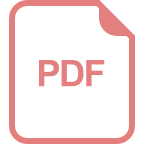
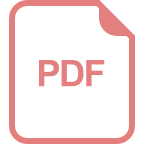
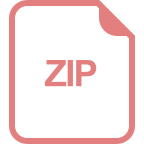
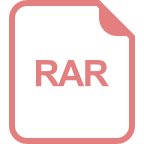
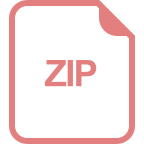
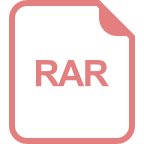
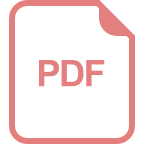
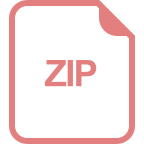
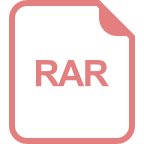
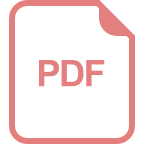
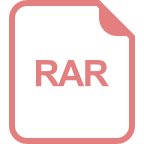