使用Requests抓取搜索python关键词页面数据
时间: 2024-10-09 15:12:29 浏览: 73
使用Python的requests库抓取搜索结果页面的数据通常涉及以下几个步骤:
1. **导入库**:首先需要导入requests库,它是Python中最常用的一个用于发送HTTP请求的工具。
```python
import requests
```
2. **设置URL**:构造你要抓取的搜索引擎搜索结果页面的URL,比如Google搜索"Python",URL可能会类似:
```python
url = "https://www.google.com/search?q=python"
```
3. **发送GET请求**:使用`requests.get()`函数向指定的URL发起GET请求,并获取响应内容。
```python
response = requests.get(url)
```
4. **检查状态码**:确保请求成功,通常200表示成功。
```python
if response.status_code == 200:
pass # 请求成功
else:
print(f"请求失败,状态码:{response.status_code}")
```
5. **解析HTML**:由于返回的是HTML格式的网页,通常需要进一步解析。可以使用如BeautifulSoup、PyQuery等库处理HTML。
6. **提取信息**:通过选择器或者其他方法从解析后的HTML中提取你需要的数据,比如搜索结果的标题、链接等。
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(response.text, 'html.parser')
results = soup.find_all('div', class_='g') # 这里假设搜索结果在名为"g"的div标签内
for result in results:
title = result.h3.a.text # 提取标题
link = result.h3.a['href'] # 提取链接
# ...其他信息提取
```
7. **保存或分析数据**:将提取的数据保存到文件或直接进行后续分析操作。
```python
with open("search_results.txt", "w") as f:
for item in data:
f.write(f"{title}\n{link}\n\n")
# 或者直接进行数据分析
analyze_data(data)
```
阅读全文
相关推荐
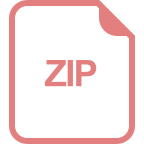
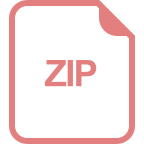
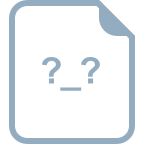















