用java语言写一个JSON格式的接口传输出去
时间: 2024-06-11 22:06:21 浏览: 97
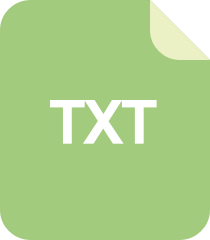
JSON在JAVA客户端和服务端传输

这里提供一个简单的Java代码示例,用于将一个Java对象转换为JSON格式,并通过HTTP POST请求将其发送到接口。
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.net.HttpURLConnection;
import java.net.URL;
import com.google.gson.Gson;
public class JsonPostExample {
public static void main(String[] args) throws Exception {
// 创建一个Java对象
MyObject obj = new MyObject("John", 25);
// 将Java对象转换为JSON字符串
Gson gson = new Gson();
String json = gson.toJson(obj);
// 设置POST请求的URL和参数
URL url = new URL("http://example.com/api");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/json");
conn.setDoOutput(true);
OutputStreamWriter writer = new OutputStreamWriter(conn.getOutputStream());
writer.write(json);
writer.flush();
// 读取响应
BufferedReader reader = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
reader.close();
writer.close();
}
// 一个简单的Java对象
private static class MyObject {
private String name;
private int age;
public MyObject(String name, int age) {
this.name = name;
this.age = age;
}
}
}
在此示例中,我们使用了Gson库将Java对象转换为JSON字符串。然后,我们设置了HTTP POST请求,将JSON字符串作为请求体发送到接口。最后,我们从响应中读取数据,以确保已成功发送请求。请注意,此示例仅供参考,实际使用时可能需要根据具体情况进行修改。
阅读全文
相关推荐
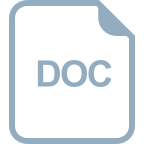
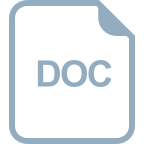
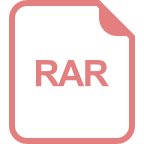
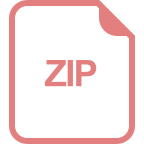
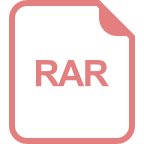
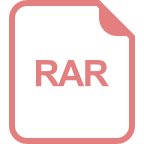
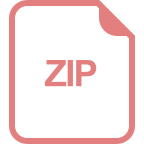
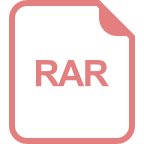
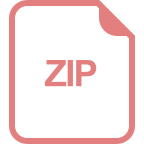
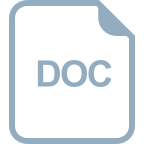
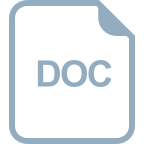
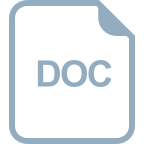
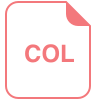
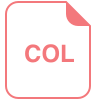
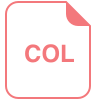
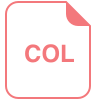
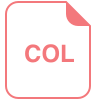
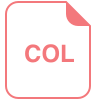