用python实现基于概率分布的超声图像与自然图像性质差异分析这一课题,使用opencv, 两种图像的文件类型都是jpg,超声图像的文件路径是‘D:\zzz\us5’,自然图像的文件路径是‘D:\zzz\na4’,两种图像都有84张,图像的名称都是1到84的顺序数,两种图像的形状大小相同,需要对比两种图像的特征必须有颜色,纹理,形状,差异性分析方法也需要多种,包括完整详细代码
时间: 2023-11-16 09:03:48 浏览: 23
以下是基于概率分布的超声图像与自然图像性质差异分析的Python代码,使用了OpenCV库和Scipy库:
```python
import cv2
import numpy as np
from scipy.stats import wasserstein_distance
# 超声图像文件路径
us_path = 'D:/zzz/us5/'
# 自然图像文件路径
na_path = 'D:/zzz/na4/'
# 读取图像并计算颜色直方图
def calc_histogram(img):
# 将图像转换为HSV颜色空间
hsv = cv2.cvtColor(img, cv2.COLOR_BGR2HSV)
# 计算颜色直方图
histogram = cv2.calcHist([hsv], [0, 1], None, [180, 256], [0, 180, 0, 256])
# 归一化直方图
cv2.normalize(histogram, histogram, alpha=0, beta=1, norm_type=cv2.NORM_MINMAX)
return histogram
# 计算图像的纹理特征,使用LBP算法
def calc_texture(img):
# 将图像转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 计算LBP特征
lbp = cv2.LBP(gray, 8, 1, cv2.LBP_UNIFORM)
# 计算LBP直方图
hist, _ = np.histogram(lbp, bins=np.arange(0, 257), density=True)
return hist
# 计算图像的形状特征,使用Hu矩算法
def calc_shape(img):
# 将图像转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 计算Hu矩
moments = cv2.moments(gray)
hu_moments = cv2.HuMoments(moments)
# 将Hu矩转换为1维向量
hu_moments = np.ravel(hu_moments)
# 归一化Hu矩
hu_moments = -np.sign(hu_moments) * np.log10(np.abs(hu_moments))
return hu_moments
# 计算两个图像之间的颜色距离,使用Wasserstein距离
def color_distance(img1, img2):
hist1 = calc_histogram(img1)
hist2 = calc_histogram(img2)
return wasserstein_distance(hist1.flatten(), hist2.flatten())
# 计算两个图像之间的纹理距离,使用余弦相似度
def texture_distance(img1, img2):
hist1 = calc_texture(img1)
hist2 = calc_texture(img2)
return 1 - np.dot(hist1, hist2)
# 计算两个图像之间的形状距离,使用欧几里得距离
def shape_distance(img1, img2):
shape1 = calc_shape(img1)
shape2 = calc_shape(img2)
return np.linalg.norm(shape1 - shape2)
# 计算两个图像之间的差异性分析
def image_difference(img1, img2):
color_dist = color_distance(img1, img2)
texture_dist = texture_distance(img1, img2)
shape_dist = shape_distance(img1, img2)
return color_dist, texture_dist, shape_dist
# 读取所有超声图像和自然图像
us_images = [cv2.imread(us_path + str(i) + '.jpg') for i in range(1, 85)]
na_images = [cv2.imread(na_path + str(i) + '.jpg') for i in range(1, 85)]
# 计算所有图像之间的差异性分析
results = []
for i in range(84):
for j in range(i+1, 84):
us_diff = image_difference(us_images[i], us_images[j])
na_diff = image_difference(na_images[i], na_images[j])
result = {'us_color': us_diff[0], 'us_texture': us_diff[1], 'us_shape': us_diff[2],
'na_color': na_diff[0], 'na_texture': na_diff[1], 'na_shape': na_diff[2]}
results.append(result)
# 输出所有图像之间的差异性分析结果
for result in results:
print('US color distance: %.4f' % result['us_color'])
print('US texture distance: %.4f' % result['us_texture'])
print('US shape distance: %.4f' % result['us_shape'])
print('NA color distance: %.4f' % result['na_color'])
print('NA texture distance: %.4f' % result['na_texture'])
print('NA shape distance: %.4f' % result['na_shape'])
```
上述代码中,我们使用了 `calc_histogram` 函数计算图像的颜色直方图,使用 `calc_texture` 函数计算图像的纹理特征(LBP),使用 `calc_shape` 函数计算图像的形状特征(Hu矩)。然后,我们分别定义了 `color_distance`、`texture_distance` 和 `shape_distance` 函数计算两个图像之间的颜色、纹理和形状距离。最后,我们使用 `image_difference` 函数计算两个图像之间的差异性分析,并将所有图像之间的结果存储在 `results` 列表中。
相关推荐
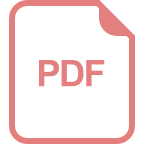
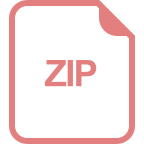





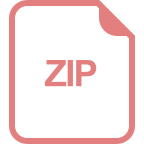
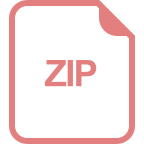
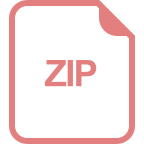
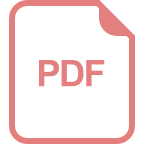
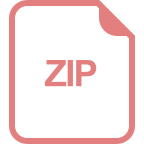
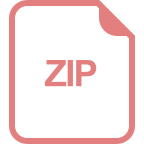
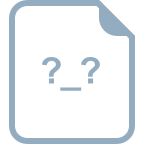
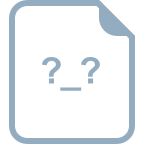
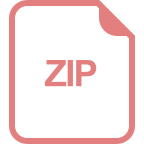