c++代码:在水电煤气管理系统中,写一个管理类,实现多人员信息录入、修改、增加、删除、以及查询系统能够找出该用户半年之内的交费记录和本次应交费的数据
时间: 2024-01-25 11:04:33 浏览: 120
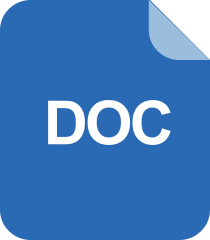
C++课程设计(公司人员管理系统)

下面是一个简单的 C++ 代码,实现了上述功能:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <ctime>
using namespace std;
// 交费记录结构体
struct PaymentRecord {
int year; // 年份
int month; // 月份
int amount; // 金额
};
// 人员信息结构体
struct PersonInfo {
string name; // 姓名
string address; // 住址
string utilityType; // 水/电/煤气
vector<PaymentRecord> records; // 交费记录
};
// 管理类
class Management {
public:
// 添加人员信息
void addPersonInfo(const PersonInfo& info) {
persons.push_back(info);
}
// 修改人员信息
void modifyPersonInfo(const string& name, const PersonInfo& info) {
for (auto& p : persons) {
if (p.name == name) {
p = info;
break;
}
}
}
// 删除人员信息
void deletePersonInfo(const string& name) {
for (auto iter = persons.begin(); iter != persons.end(); ++iter) {
if (iter->name == name) {
persons.erase(iter);
break;
}
}
}
// 查询人员信息
vector<PersonInfo> queryPersonInfo(const string& name) const {
vector<PersonInfo> result;
for (const auto& p : persons) {
if (p.name == name) {
result.push_back(p);
}
}
return result;
}
// 查询半年交费记录
vector<PaymentRecord> queryPaymentRecord(const string& name) const {
vector<PaymentRecord> result;
const time_t now = time(nullptr); // 获取当前时间
for (const auto& p : persons) {
if (p.name == name) {
for (const auto& r : p.records) {
const time_t recordTime = mktime(&tm{r.year - 1900, r.month - 1, 0, 0, 0, 0, 0, 0, 0});
if (now - recordTime <= 6 * 30 * 24 * 60 * 60) {
result.push_back(r);
}
}
break;
}
}
return result;
}
// 计算本次应交费的数据
int calculatePaymentAmount(const string& name) const {
const int price = getPriceByUtilityType(name);
const time_t now = time(nullptr); // 获取当前时间
const tm* timeinfo = localtime(&now);
const int year = timeinfo->tm_year + 1900;
const int month = timeinfo->tm_mon + 1;
for (const auto& p : persons) {
if (p.name == name) {
for (const auto& r : p.records) {
if (r.year == year && r.month == month) {
// 本月已经交过费了
return -1;
}
}
// 本月还未交费,计算应交费用
return price;
}
}
return -1;
}
private:
// 根据用水/电/煤气类型获取价格
int getPriceByUtilityType(const string& utilityType) const {
if (utilityType == "水") {
return 50;
} else if (utilityType == "电") {
return 100;
} else if (utilityType == "煤气") {
return 80;
}
return 0;
}
vector<PersonInfo> persons; // 所有人员信息
};
int main() {
Management management;
// 添加人员信息
management.addPersonInfo({"张三", "北京市海淀区", "水", {{2021, 3, 50}, {2021, 4, 50}, {2021, 5, 50}, {2021, 6, 50}, {2021, 7, 50}}});
management.addPersonInfo({"李四", "北京市朝阳区", "电", {{2021, 3, 100}, {2021, 4, 100}, {2021, 5, 100}, {2021, 6, 100}, {2021, 7, 100}}});
management.addPersonInfo({"王五", "北京市东城区", "煤气", {{2021, 3, 80}, {2021, 4, 80}, {2021, 5, 80}, {2021, 6, 80}, {2021, 7, 80}}});
// 查询人员信息
auto result = management.queryPersonInfo("张三");
for (const auto& r : result) {
cout << "姓名:" << r.name << endl;
cout << "住址:" << r.address << endl;
cout << "用水/电/煤气类型:" << r.utilityType << endl;
cout << "交费记录:" << endl;
for (const auto& p : r.records) {
cout << p.year << "年" << p.month << "月:" << p.amount << "元" << endl;
}
cout << endl;
}
// 查询半年交费记录
result.clear();
result = management.queryPaymentRecord("张三");
cout << "张三半年交费记录:" << endl;
for (const auto& r : result) {
cout << r.year << "年" << r.month << "月:" << r.amount << "元" << endl;
}
cout << endl;
// 计算本次应交费的数据
const int amount = management.calculatePaymentAmount("张三");
if (amount < 0) {
cout << "张三本月已交过费" << endl;
} else {
cout << "张三本月应交费:" << amount << "元" << endl;
}
// 修改人员信息
management.modifyPersonInfo("王五", {"王五", "北京市西城区", "煤气", {{2021, 3, 80}, {2021, 4, 80}, {2021, 5, 80}, {2021, 6, 80}, {2021, 7, 80}, {2021, 8, 80}}});
// 删除人员信息
management.deletePersonInfo("李四");
return 0;
}
```
上面的代码中,我们定义了两个结构体 `PaymentRecord` 和 `PersonInfo`,分别用于存储交费记录和人员信息。然后,我们定义了一个 `Management` 类,用于管理人员信息和交费记录。该类包含了添加、修改、删除、查询人员信息的操作,以及查询半年交费记录和计算本次应交费的操作。
在 `Management` 类中,我们使用了 `vector` 来存储所有人员信息,使用了 `time` 和 `mktime` 函数来计算时间差和将年月日转换为时间戳。在 `calculatePaymentAmount` 函数中,我们根据当前时间和已有的交费记录来计算本次应交费的数据。
最后,在 `main` 函数中,我们演示了如何使用 `Management` 类进行人员信息的添加、修改、删除、查询,以及查询半年交费记录和计算本次应交费的数据。
阅读全文
相关推荐
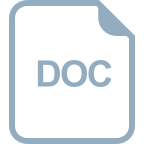
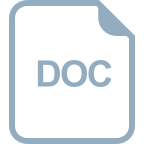
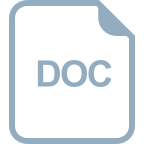
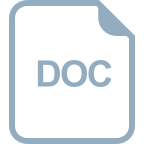
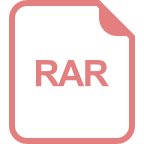
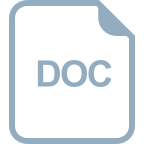
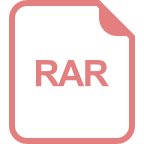
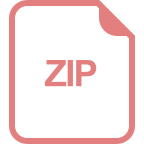
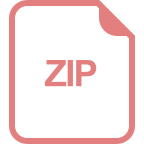
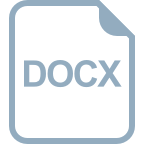
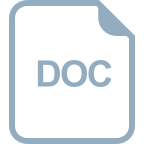
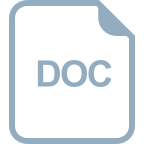
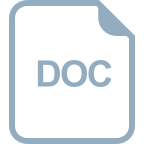
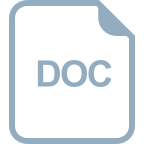
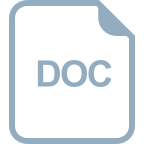
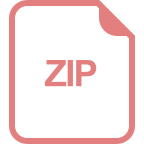
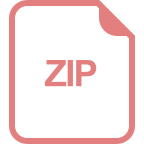
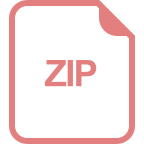