#include<iostream> #include<stack> #include<string> #include<cctype> #include<sstream> using namespace std; stack< long long >sk; string str; stringstream tempIO; long long Calculate( long a, long b, char op) { switch( op ) { case '+': return a + b; case '-': return a - b; case '*': return a * b; case '/': return a / b; } } int main() { getline( cin,str ); for(int i=0; str[i]!='@'; i++){ if( isdigit(str[i] ) ){ tempIO << str[i]; }else if(str[i]==' '){ long long = 0; tempIO >> num; sk.push( num ); tempIO.clear(); } else { long long t1= sk.top(); sk.pop(); long long t2 = sk.top(); sk.pop(); sk.push( Calculate( t2, t1, str[i] )); } } cout << sk.top() << endl; return 0; }
时间: 2024-02-14 09:30:08 浏览: 47
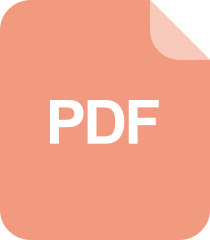
C++常用的#include头文件总结
在您提供的代码中,有一个问题需要注意:
```cpp
else if(str[i]==' '){
long long = 0;
tempIO >> num;
sk.push( num );
tempIO.clear();
}
```
在这段代码中,您定义了一个名为 `long long` 的变量,但是没有为其指定一个变量名。这会导致编译错误。您需要为该变量指定一个名称,例如:
```cpp
else if(str[i]==' '){
long long num = 0; // 给变量指定一个名称,例如 num
tempIO >> num;
sk.push( num );
tempIO.clear();
}
```
修复此问题后,您的代码应该可以正常编译和运行了。
阅读全文
相关推荐
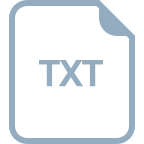
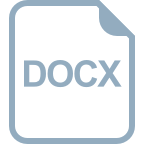









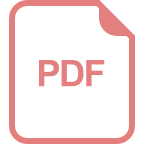
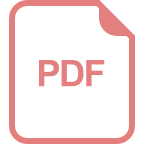
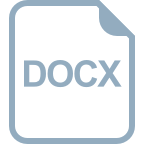
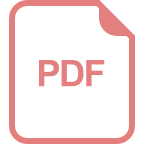
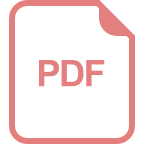
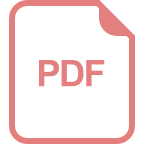