请你作为java开发程序员实现优惠卷功能
时间: 2024-09-08 10:03:42 浏览: 40
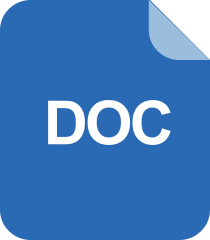
Java程序员面试简历.doc
在Java中实现优惠券功能,首先需要定义一个优惠券类(Coupon),其中包含优惠券的基本属性和行为。以下是一个简单的示例:
```java
// 优惠券类
public class Coupon {
// 优惠券属性
private String id; // 优惠券编号
private String name; // 优惠券名称
private double discount; // 折扣或优惠金额
private Date startTime; // 优惠券开始时间
private Date endTime; // 优惠券结束时间
private int usageLimit; // 使用限制,比如每人限用1次
private int usedTimes; // 已使用次数
// 构造方法
public Coupon(String id, String name, double discount, Date startTime, Date endTime, int usageLimit) {
this.id = id;
this.name = name;
this.discount = discount;
this.startTime = startTime;
this.endTime = endTime;
this.usageLimit = usageLimit;
this.usedTimes = 0; // 初始使用次数为0
}
// 其他getter和setter方法...
// 判断优惠券是否有效
public boolean isValid() {
Date now = new Date();
return !now.before(startTime) && !now.after(endTime);
}
// 判断是否达到使用限制
public boolean canUse() {
return usedTimes < usageLimit;
}
// 使用优惠券,增加已使用次数
public void useCoupon() {
if (canUse()) {
usedTimes++;
} else {
throw new IllegalStateException("已经达到了使用限制");
}
}
}
// 优惠券的使用示例
public class CouponDemo {
public static void main(String[] args) {
// 创建优惠券实例
Coupon coupon = new Coupon("001", "满100减20", 20.0, new Date(System.currentTimeMillis() - 1000L), new Date(System.currentTimeMillis() + 86400000L), 1);
// 检查优惠券是否有效
if (coupon.isValid()) {
try {
// 使用优惠券
coupon.useCoupon();
System.out.println("优惠券使用成功!");
} catch (IllegalStateException e) {
System.out.println(e.getMessage());
}
} else {
System.out.println("优惠券已过期,无法使用!");
}
}
}
```
在上述代码中,`Coupon` 类包含了优惠券的编号、名称、折扣、时间限制以及使用次数等属性。同时提供了判断优惠券是否有效、是否可使用和使用优惠券的方法。`CouponDemo` 类中的 `main` 方法则是优惠券使用的一个简单示例。
阅读全文
相关推荐
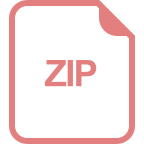
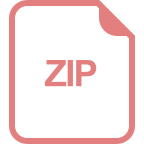















