给出圆柱底面圆的圆心坐标和半径以及圆柱的高,绘制3维圆柱图形 winform c# GDI+
时间: 2024-09-25 16:05:48 浏览: 7
在WinForms C#中使用GDI+(Graphics Device Interface Plus)来绘制3D圆柱体,你需要使用System.Drawing命名空间下的几个关键类,如Graphics、RectangleF等,并利用Transformations类中的Matrix变形操作来模拟3D效果。以下是一个基本的示例:
```csharp
using System.Drawing;
using System.Windows.Forms;
public class CustomDrawForm : Form
{
private Graphics graphics;
private Pen pen;
private SolidBrush brush;
public CustomDrawForm()
{
// 初始化笔和刷
pen = new Pen(Color.Black, 2);
brush = new SolidBrush(Color.Gray);
// 设置绘图区域
this.Size = new Size(500, 500);
this.Paint += Draw3DCylinder;
}
// 画3D圆柱函数
private void Draw3DCylinder(object sender, PaintEventArgs e)
{
graphics = e.Graphics;
// 圆柱参数
PointF bottomCenter = new PointF(100, 100); // 底部圆心坐标
float radius = 50; // 半径
float height = 200; // 高度
// 创建圆底面
RectangleF cylinderBase = new RectangleF(bottomCenter.X - radius, bottomCenter.Y - radius, radius * 2, radius * 2);
graphics.FillEllipse(brush, cylinderBase);
// 创建透视变换矩阵
Matrix matrix = new Matrix();
matrix.Translate(0, 0, height / 4); // 向上移动一段距离模拟3D
matrix.RotateAt(Math.PI / 4, bottomCenter); // 旋转45度
// 使用透视变换
graphics.PushTransform(matrix);
// 绘制圆柱侧面(矩形)
graphics.DrawRectangle(pen, cylinderBase.Left, cylinderBase.Top, cylinderBase.Width, cylinderBase.Height);
graphics.PopTransform(); // 恢复原视角
}
protected override void OnPaint(PaintEventArgs e) => Draw3DCylinder(sender, e);
}
// 在Main函数中创建并显示这个窗体
[STAThread]
static void Main()
{
Application.Run(new CustomDrawForm());
}
```
这只是一个基础示例,实际的3D效果可能需要结合Z轴和阴影效果,以及更复杂的变换。如果你想要完全模拟真实3D效果,可能需要使用专门处理3D图形的库,如OpenGL或DirectX。
相关推荐
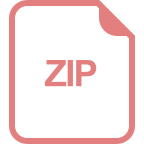
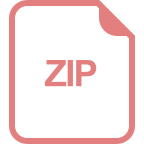
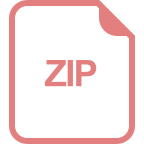














