c++栈判断运算符优先级
时间: 2023-11-20 07:50:34 浏览: 87
在C++中,可以使用栈来判断运算符的优先级。具体实现方法是,将运算符依次压入栈中,每次压入运算符时,先判断栈顶运算符的优先级是否高于当前运算符,如果是,则将栈顶运算符弹出并进行运算,直到栈顶运算符的优先级低于或等于当前运算符的优先级,然后将当前运算符压入栈中。最后,将栈中剩余的运算符依次弹出并进行运算。
下面是一个简单的示例代码:
```c++
#include <iostream>
#include <stack>
using namespace std;
int priority(char op) {
if (op == '+' || op == '-') return 1;
if (op == '*' || op == '/') return 2;
return 0;
}
int main() {
string expr = "3+4*5-(6+7)*8";
stack<char> opStack;
string postfixExpr = "";
for (int i = 0; i < expr.length(); i++) {
char ch = expr[i];
if (isdigit(ch)) {
postfixExpr += ch;
} else if (ch == '(') {
opStack.push(ch);
} else if (ch == ')') {
while (!opStack.empty() && opStack.top() != '(') {
postfixExpr += opStack.top();
opStack.pop();
}
opStack.pop();
} else {
while (!opStack.empty() && priority(opStack.top()) >= priority(ch)) {
postfixExpr += opStack.top();
opStack.pop();
}
opStack.push(ch);
}
}
while (!opStack.empty()) {
postfixExpr += opStack.top();
opStack.pop();
}
cout << postfixExpr << endl;
return 0;
}
```
输出结果为:`345*+67+8*-`
相关推荐
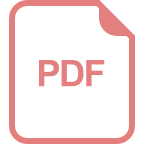














