springboot写一个使用easyexcel进行读取本地excel文件内容并将读到的内容返回给前端的api
时间: 2024-09-15 21:03:57 浏览: 89
Spring Boot 中使用 EasyExcel 进行读取本地 Excel 文件并将其内容作为 API 返回的过程可以分为以下几个步骤:
1. 添加依赖:
首先,在你的 `pom.xml` 或者 Gradle 的 build 文件中添加 EasyExcel 和 Springfox Swagger 相关的依赖:
```xml
<!-- Maven -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>easyexcel</artifactId>
<version>最新版本号</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>最新版本号</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>最新版本号</version>
</dependency>
<!-- Gradle (如果使用的是Gradle) -->
implementation 'com.alibaba:easyexcel:最新版本号'
implementation 'io.springfox:springfox-swagger2:最新版本号'
implementation 'io.springfox:springfox-swagger-ui:最新版本号'
```
2. 创建数据模型:
定义一个 Java 对象,映射 Excel 的表头,例如:
```java
public class SheetData {
private String columnName1;
private String columnName2;
// ...其他列
// getters and setters
}
```
3. Excel 读取处理器:
使用 EasyExcel 的 `@ControllerAdvice` 注解创建一个处理 Excel 读取的类:
```java
@RestControllerAdvice
public class ExcelControllerAdvice {
@PostMapping("/read-excel")
@ApiOperation("读取Excel内容")
public ResponseEntity<List<SheetData>> readExcel(@RequestParam("file") MultipartFile file) throws IOException {
List<SheetData> dataList = new ArrayList<>();
try (InputStream inputStream = file.getInputStream()) {
EasyExcel.read(inputStream, SheetData.class)
.doRead(dataList);
}
return ResponseEntity.ok(dataList);
}
}
```
这里假设上传的文件名为 `file`。
4. 接口文档:
使用 Springfox 提供了注解如 `@ApiOperation`, `@ApiParam` 来描述 API 的功能和参数,方便生成文档。
5. 测试和部署:
启动 Spring Boot 应用,通过 POST 请求 `/read-excel` 并上传 Excel 文件,服务器会读取内容并返回给前端。
**相关问题--:**
1. 在使用EasyExcel时,如何处理异常?
2. 如何将读取结果转换成适合前端展示的数据结构?
3. 如果需要支持多种格式的文件,EasyExcel如何配置?
阅读全文
相关推荐
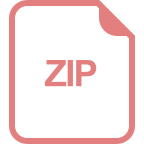
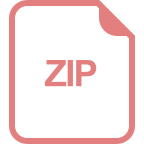
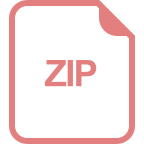
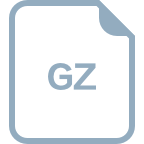
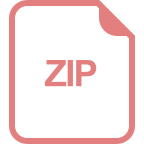
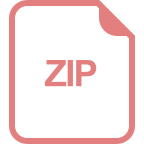
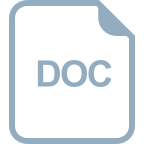
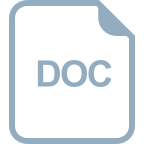
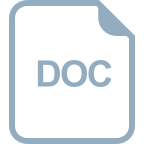
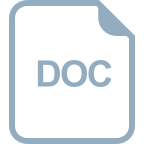




