使用C语言编程完成以下功能 用pthread_create创建一 个线程并 显示你的名字+学号; 主线程计算0 ~ 20之间被4整除的数之和并显示。
时间: 2024-11-20 17:38:58 浏览: 5
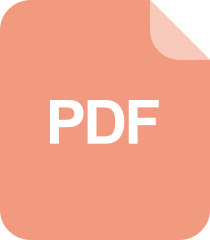
linux创建线程之pthread_create的具体使用

在C语言中,你可以使用POSIX线程库(pthread.h)来创建一个新的线程,并利用printf函数显示消息。首先,确保已经包含必要的头文件`stdio.h`和`pthread.h`。
下面是一个简单的示例程序,展示了如何创建一个新线程并同时在主线程中计算4的倍数之和:
```c
#include <stdio.h>
#include <pthread.h>
// 线程回调函数
void* display_info(void *arg) {
char name[] = "Your Name"; // 替换为实际的名字
int student_id = 1234; // 替换为实际的学生ID
printf("Thread started by %s with ID: %d\n", name, student_id);
return NULL;
}
// 主线程函数
void compute_sum() {
int sum = 0;
for (int i = 0; i <= 20; i++) {
if (i % 4 == 0) {
sum += i;
}
}
printf("The sum of numbers divisible by 4 from 0 to 20 is: %d\n", sum);
}
int main() {
pthread_t thread_id; // 创建线程标识符
// 创建新的线程并传递回调函数
pthread_create(&thread_id, NULL, display_info, NULL);
// 计算和显示结果
compute_sum();
// 等待新线程结束
pthread_join(thread_id, NULL); // 如果不想阻塞,可以使用pthread_detach()
return 0;
}
```
在这个程序中,我们首先定义了一个名为`display_info`的回调函数,这个函数将在新线程中运行。然后,在`main`函数里,我们创建了新线程并将`display_info`作为线程的目标函数。接着,主线程执行`compute_sum`来计算4的倍数之和。最后,我们使用`pthread_join`等待新线程结束。
阅读全文
相关推荐
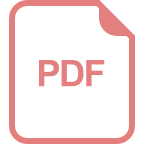
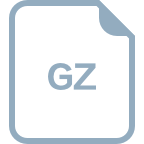


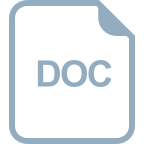












