python爬取网页多页表格数据快速
时间: 2024-09-30 20:14:32 浏览: 12
在Python中,可以使用BeautifulSoup、pandas和requests等库组合起来高效地爬取网页多页表格数据。以下是基本步骤:
1. **安装所需库**:
首先需要安装`beautifulsoup4`, `lxml`(BeautifulSoup的一个解析器),以及`pandas`。你可以使用pip安装:
```
pip install beautifulsoup4 lxml pandas requests
```
2. **获取网页内容**:
使用`requests.get()`函数从目标网址获取HTML源码,例如:
```python
import requests
url = 'http://example.com/page1'
response = requests.get(url)
html_content = response.text
```
3. **解析HTML**:
使用BeautifulSoup解析HTML内容,找到包含表格的部分:
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'lxml')
table = soup.find('table') # 根据实际页面结构选择合适的标签
```
4. **提取表格数据**:
使用BeautifulSoup的`.find_all()`方法提取表格行(tr)和列(td或th),然后转换成pandas DataFrame:
```python
rows = table.find_all('tr')
data_list = []
for row in rows:
cols = row.find_all(['td', 'th'])
cols_data = [col.text.strip() for col in cols]
data_list.append(cols_data)
df = pd.DataFrame(data_list)
```
5. **处理分页**:
如果是多页数据,通常会在URL中包含页码参数。你需要遍历所有页数并调整URL来获取每一页的内容。例如,如果每页的URL模式是`http://example.com/page/<page_number>`,你可以用循环和切片来实现:
```python
base_url = 'http://example.com/page/'
pages = range(1, n_pages + 1) # 确定总页数n_pages
dfs = [] # 用于存储所有页面的数据
for page_num in pages:
url = base_url + str(page_num)
response = requests.get(url)
... # 这里重复解析步骤直到将所有页面的数据合并到dfs列表中
all_data = pd.concat(dfs)
```
6. **合并数据**:
将所有单页DataFrame合并成一个大表。
7. **清理数据**:
检查数据清洗,如删除空值、异常值等。
相关推荐
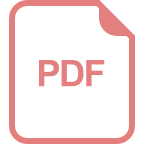
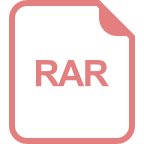
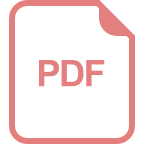














