结合Jsoup, apache tika, lucene等来搭建搜索引擎,能够处理多种格式的文档(如PDF、Word、HTML等),并允许用户根据自己的需求进行搜索。若采用SpringTools进行开发,请为我实现上述要求,提供整体的框架,包括包名,类名,以及所有需要的代码
时间: 2024-12-16 16:24:38 浏览: 4
在Spring Tools中构建一个结合了Jsoup、Apache Tika和Lucene的搜索引擎,主要步骤会涉及以下几个关键部分:
1. **包结构**:
- `com.example.searchengine`: 主要应用包,包含核心组件和服务。
- `com.example.searchengine.service`: 服务层,存放数据处理和搜索相关的类。
- `com.example.searchengine.repository`: 数据访问层,处理数据库或文件存储。
- `com.example.searchengine.web`: 控制层和Web界面。
2. **类名称示例**:
- `DocumentConverterService` (com.example.searchengine.service): 使用Tika转换各种文档格式。
- `DocumentIndexer` (com.example.searchengine.service): 将文本内容索引到Lucene库。
- `SearchEngineRepository` (com.example.searchengine.repository): Lucene Index Manager.
- `SearchController` (com.example.searchengine.web): 接受用户请求并调用搜索服务。
以下是简化的代码片段:
```java
// DocumentConverterService.java
package com.example.searchengine.service;
import org.apache.tika.Tika;
import org.apache.tika.metadata.Metadata;
import org.apache.tika.parser.ParseContext;
import org.apache.tika.parser.Parser;
import org.springframework.stereotype.Service;
import org.springframework.util.FileCopyUtils;
@Service
public class DocumentConverterService {
private final Parser parser = new Tika();
public String convertToText(String filePath) throws Exception {
ParseContext context = new ParseContext();
return FileCopyUtils.copyToByteArray(parser.parse(filePath, Metadata.get(), context)).toString("UTF-8");
}
}
// SearchEngineRepository.java
package com.example.searchengine.repository;
import org.apache.lucene.document.Document;
import org.apache.lucene.index.DirectoryReader;
import org.apache.lucene.queryparser.classic.QueryParser;
import org.apache.lucene.search.IndexSearcher;
import org.apache.lucene.store.Directory;
public interface SearchEngineRepository {
void indexDocument(Document document);
QuerySearcher createSearcher();
}
// SearchController.java
package com.example.searchengine.web;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import com.example.searchengine.service.SearchEngineRepository;
@RestController
public class SearchController {
@Autowired
private SearchEngineRepository searchRepository;
@GetMapping("/search")
public ResponseEntity<String> search(@RequestParam String query) {
IndexSearcher searcher = searchRepository.createSearcher();
// 实现查询逻辑...
return ResponseEntity.ok(result);
}
}
```
注意这只是一个简化版的基础框架,实际项目中还需要处理文件读取错误、分词索引、结果排序和显示等更多细节。同时,为了更高效地操作,可以考虑使用Elasticsearch或其他专门的全文检索库代替Lucene。
阅读全文
相关推荐
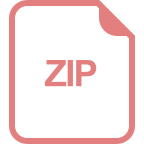
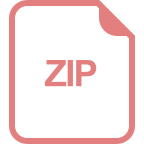
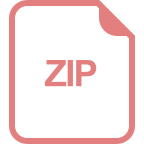
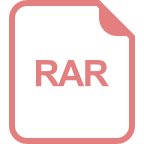
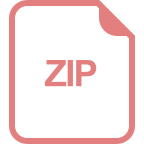
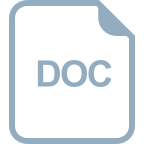
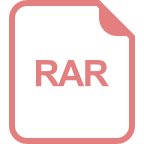
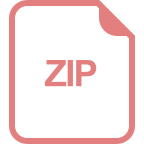
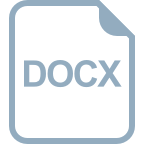
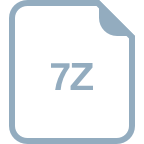
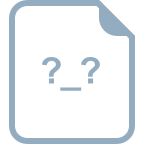
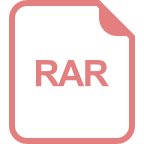
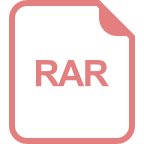
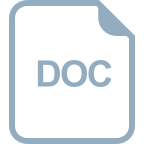
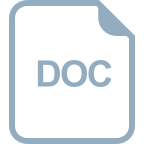