PCL实现对布尔描述子特征匹配并将匹配结果可视化
时间: 2024-02-13 12:03:29 浏览: 25
PCL中提供了实现布尔描述子特征匹配的相关函数和类,下面是一个简单的示例代码:
```cpp
#include <pcl/point_cloud.h>
#include <pcl/point_types.h>
#include <pcl/features/shot_omp.h>
#include <pcl/registration/correspondence_estimation.h>
#include <pcl/visualization/pcl_visualizer.h>
using namespace pcl;
typedef PointXYZRGBNormal PointT;
typedef Histogram<352> FeatureT;
int main()
{
// 读取点云数据
PointCloud<PointT>::Ptr cloud1(new PointCloud<PointT>);
PointCloud<PointT>::Ptr cloud2(new PointCloud<PointT>);
// ... 读取数据代码
// 计算SHOT特征
FeatureCloud<FeatureT>::Ptr features1(new FeatureCloud<FeatureT>);
FeatureCloud<FeatureT>::Ptr features2(new FeatureCloud<FeatureT>);
SHOTEstimationOMP<PointT, Normal, FeatureT> shot;
search::KdTree<PointT>::Ptr tree(new search::KdTree<PointT>());
shot.setSearchMethod(tree);
shot.setRadiusSearch(0.02);
shot.setInputCloud(cloud1);
shot.setInputNormals(cloud1);
shot.compute(*features1);
shot.setInputCloud(cloud2);
shot.setInputNormals(cloud2);
shot.compute(*features2);
// 计算匹配点对
CorrespondencesPtr correspondences(new Correspondences());
CorrEstimation<FeatureT, FeatureT> corr;
corr.setInputSource(features1);
corr.setInputTarget(features2);
corr.determineCorrespondences(*correspondences);
// 可视化匹配结果
PCLVisualizer viewer;
viewer.addPointCloud(cloud1, "cloud1");
viewer.addPointCloud(cloud2, "cloud2");
for (size_t i = 0; i < correspondences->size(); ++i) {
PointT p1 = cloud1->points[correspondences->at(i).index_query];
PointT p2 = cloud2->points[correspondences->at(i).index_match];
std::stringstream ss;
ss << "line_" << i;
viewer.addLine(p1, p2, 1.0, 0.0, 0.0, ss.str());
}
while (!viewer.wasStopped()) {
viewer.spinOnce();
}
return 0;
}
```
以上代码中,我们首先读取了两个点云数据,并使用SHOT特征计算了它们的布尔描述子。然后,我们使用`CorrEstimation`类计算了匹配点对,最后将匹配结果可视化。
需要注意的是,PCL中提供了多种布尔描述子类型和匹配算法,具体的实现代码可能略有不同。此外,为了方便演示,上述代码中省略了一些错误处理和参数调整等步骤,实际使用时需要根据实际情况进行调整。
相关推荐
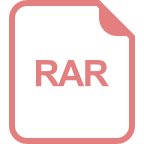














