Kotlin/JS 来编写 JavaScript 用XMLHttpRequest發送GET和POST請求
时间: 2023-06-17 21:05:21 浏览: 52
在Kotlin/JS中,可以使用JavaScript的原生XMLHttpRequest对象来发送GET和POST请求。以下是一个简单的示例:
首先,需要在Gradle中添加以下依赖:
```groovy
implementation 'org.jetbrains.kotlin:kotlin-stdlib-js'
```
然后,可以编写一个包含发送GET和POST请求的类:
```kotlin
import org.w3c.xhr.XMLHttpRequest
import kotlin.js.json
class Http {
companion object {
fun get(url: String, callback: (String) -> Unit) {
val xhr = XMLHttpRequest()
xhr.open("GET", url)
xhr.onload = {
if (xhr.status == 200.toShort()) {
callback(xhr.responseText)
} else {
// handle error
}
}
xhr.send()
}
fun post(url: String, data: Map<String, String>, callback: (String) -> Unit) {
val xhr = XMLHttpRequest()
xhr.open("POST", url)
xhr.setRequestHeader("Content-Type", "application/json")
xhr.onload = {
if (xhr.status == 200.toShort()) {
callback(xhr.responseText)
} else {
// handle error
}
}
xhr.send(JSON.stringify(data))
}
}
}
```
这个类包含两个静态方法:`get`和`post`。这些方法接受一个URL和一个回调函数。在回调函数中,可以处理服务器响应。
要发送GET请求,可以使用以下代码:
```kotlin
Http.get("https://example.com/api/data", { response ->
console.log(response)
})
```
要发送POST请求,可以使用以下代码:
```kotlin
val data = mapOf("name" to "John", "age" to "30")
Http.post("https://example.com/api/data", data, { response ->
console.log(response)
})
```
在这个示例中,POST请求发送了一个包含"name"和"age"字段的JSON对象。服务器可以使用这些值来处理请求。
相关推荐
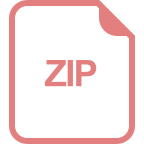














