使用C# 设计一个excel文件转换多种格式的程序
时间: 2024-02-10 22:10:25 浏览: 150
好的,下面是一个简单的 C# 程序,可以将 Excel 文件转换为多种格式,如 CSV、XML 和 JSON 等。程序使用了 Microsoft.Office.Interop.Excel 库来读取 Excel 文件,使用 Newtonsoft.Json 库来序列化为 JSON 格式。
```csharp
using System;
using System.IO;
using System.Linq;
using Microsoft.Office.Interop.Excel;
using Newtonsoft.Json;
namespace ExcelConverter
{
class Program
{
static void Main(string[] args)
{
if (args.Length < 2)
{
Console.WriteLine("Usage: ExcelConverter.exe <input_file> <output_format>");
return;
}
string inputFile = args[0];
string outputFormat = args[1];
Application excelApp = new Application();
Workbook workbook = excelApp.Workbooks.Open(inputFile);
Worksheet worksheet = workbook.ActiveSheet;
Range range = worksheet.UsedRange;
switch (outputFormat)
{
case "csv":
ConvertToCsv(range);
break;
case "xml":
ConvertToXml(range);
break;
case "json":
ConvertToJson(range);
break;
default:
Console.WriteLine($"Unsupported output format: {outputFormat}");
break;
}
workbook.Close();
excelApp.Quit();
}
static void ConvertToCsv(Range range)
{
string csv = "";
for (int row = 1; row <= range.Rows.Count; row++)
{
string[] values = Enumerable.Range(1, range.Columns.Count)
.Select(col => range.Cells[row, col].Value.ToString())
.ToArray();
csv += string.Join(",", values) + Environment.NewLine;
}
Console.WriteLine(csv);
}
static void ConvertToXml(Range range)
{
string xml = "<?xml version=\"1.0\" encoding=\"UTF-8\"?>" + Environment.NewLine;
xml += "<table>" + Environment.NewLine;
for (int row = 1; row <= range.Rows.Count; row++)
{
xml += "<row>" + Environment.NewLine;
for (int col = 1; col <= range.Columns.Count; col++)
{
string cellValue = range.Cells[row, col].Value.ToString();
xml += $"<col{col}>{cellValue}</col{col}>" + Environment.NewLine;
}
xml += "</row>" + Environment.NewLine;
}
xml += "</table>" + Environment.NewLine;
Console.WriteLine(xml);
}
static void ConvertToJson(Range range)
{
dynamic table = new System.Dynamic.ExpandoObject();
table.rows = Enumerable.Range(1, range.Rows.Count)
.Select(row => {
dynamic rowData = new System.Dynamic.ExpandoObject();
for (int col = 1; col <= range.Columns.Count; col++)
{
string colName = $"col{col}";
string cellValue = range.Cells[row, col].Value.ToString();
((IDictionary<string, object>)rowData)[colName] = cellValue;
}
return rowData;
})
.ToList();
string json = JsonConvert.SerializeObject(table, Formatting.Indented);
Console.WriteLine(json);
}
}
}
```
该程序使用命令行参数来指定输入文件和输出格式,例如:
```
ExcelConverter.exe input.xlsx csv
```
在上述示例中,程序将 `input.xlsx` 文件转换为 CSV 格式,并将结果输出到控制台。你可以根据需要修改程序代码,以支持更多的输出格式和选项。
阅读全文
相关推荐
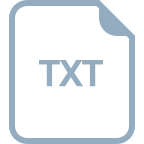
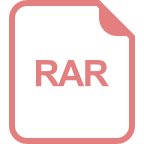
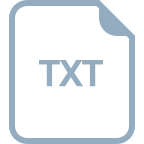
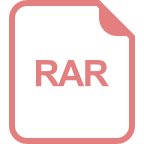
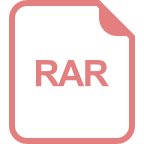
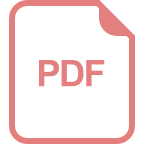
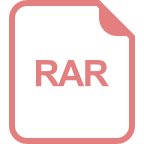
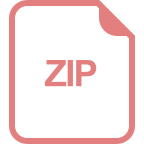
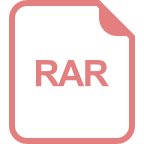
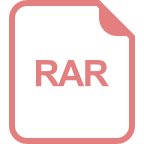
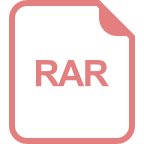
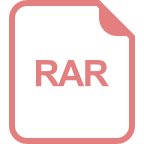
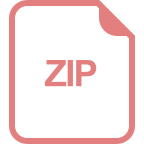
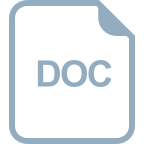
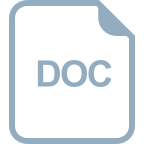
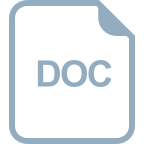