编程实现进程间管道通信。要求:父子进程共享一无名管道,两个子进程作为发送方分别向管道发送一行信息,父进程先读出子进程P1发来的信息行,将其转换为大写字母输出;再读出子进程P2发来的信息行,将其转换为小写字母输出。
时间: 2023-12-30 20:02:57 浏览: 46
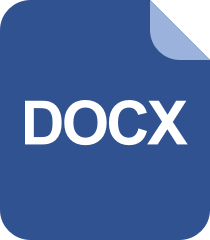
进程间通信之管道通信
下面是用 C 语言实现的进程间管道通信的代码:
```c
#include <stdio.h>
#include <unistd.h>
#include <string.h>
#include <ctype.h>
#define BUFFER_SIZE 32
int main()
{
int pipe_fd[2];
pid_t pid;
char buffer[BUFFER_SIZE];
if (pipe(pipe_fd) < 0) {
printf("Failed to create pipe.\n");
return -1;
}
pid = fork();
if (pid < 0) {
printf("Failed to create child process.\n");
return -1;
}
else if (pid == 0) { // P1
close(pipe_fd[0]); // 关闭读端口
char *msg = "Hello, I'm P1.";
write(pipe_fd[1], msg, strlen(msg)+1); // 发送信息到管道
close(pipe_fd[1]); // 关闭写端口
}
else { // 父进程
pid = fork();
if (pid < 0) {
printf("Failed to create child process.\n");
return -1;
}
else if (pid == 0) { // P2
close(pipe_fd[0]); // 关闭读端口
char *msg = "Hello, I'm P2.";
write(pipe_fd[1], msg, strlen(msg)+1); // 发送信息到管道
close(pipe_fd[1]); // 关闭写端口
}
else { // 父进程
close(pipe_fd[1]); // 关闭写端口
read(pipe_fd[0], buffer, BUFFER_SIZE); // 读取 P1 发来的信息
printf("Received from P1: %s\n", buffer);
for (int i = 0; i < strlen(buffer); i++) {
buffer[i] = toupper(buffer[i]); // 转换为大写字母
}
printf("Converted to upper case: %s\n", buffer);
read(pipe_fd[0], buffer, BUFFER_SIZE); // 读取 P2 发来的信息
printf("Received from P2: %s\n", buffer);
for (int i = 0; i < strlen(buffer); i++) {
buffer[i] = tolower(buffer[i]); // 转换为小写字母
}
printf("Converted to lower case: %s\n", buffer);
close(pipe_fd[0]); // 关闭读端口
}
}
return 0;
}
```
这个程序创建了一个无名管道,然后通过 fork() 函数创建了两个子进程 P1 和 P2,它们分别向管道发送一行信息。父进程从管道中读取这两行信息,并分别将它们转换为大写字母和小写字母输出。注意,父进程需要先关闭管道的写端口,然后再读取管道中的数据,否则读取操作将会一直阻塞等待。
阅读全文
相关推荐
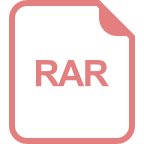
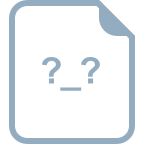
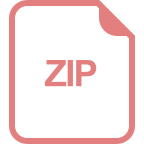
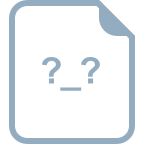
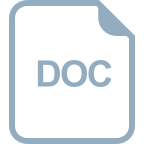
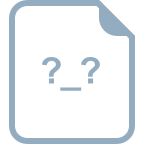
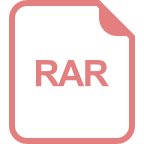
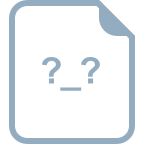
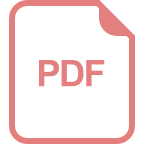
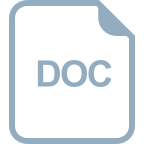
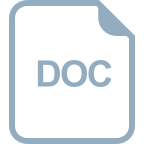
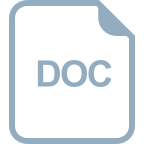
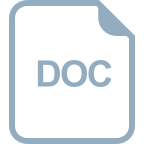
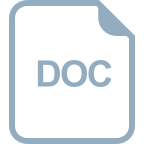
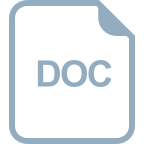
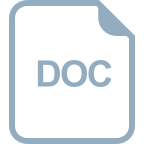
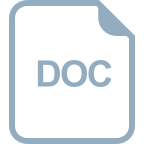