springboot配置多个rabbitmq
时间: 2023-05-31 15:20:45 浏览: 217
### 回答1:
Spring Boot可以很容易地配置多个RabbitMQ实例。以下是配置多个RabbitMQ实例的步骤:
1. 在application.properties文件中添加多个RabbitMQ实例的配置信息。例如:
spring.rabbitmq.host=host1
spring.rabbitmq.port=5672
spring.rabbitmq.username=user1
spring.rabbitmq.password=password1
spring.rabbitmq.second.host=host2
spring.rabbitmq.second.port=5672
spring.rabbitmq.second.username=user2
spring.rabbitmq.second.password=password2
2. 创建多个RabbitTemplate和ConnectionFactory bean。例如:
@Configuration
public class RabbitMQConfig {
@Bean
public ConnectionFactory connectionFactory() {
CachingConnectionFactory connectionFactory = new CachingConnectionFactory();
connectionFactory.setHost(env.getProperty("spring.rabbitmq.host"));
connectionFactory.setPort(env.getProperty("spring.rabbitmq.port", Integer.class));
connectionFactory.setUsername(env.getProperty("spring.rabbitmq.username"));
connectionFactory.setPassword(env.getProperty("spring.rabbitmq.password"));
return connectionFactory;
}
@Bean
public RabbitTemplate rabbitTemplate() {
RabbitTemplate template = new RabbitTemplate(connectionFactory());
return template;
}
@Bean(name = "secondConnectionFactory")
public ConnectionFactory secondConnectionFactory() {
CachingConnectionFactory connectionFactory = new CachingConnectionFactory();
connectionFactory.setHost(env.getProperty("spring.rabbitmq.second.host"));
connectionFactory.setPort(env.getProperty("spring.rabbitmq.second.port", Integer.class));
connectionFactory.setUsername(env.getProperty("spring.rabbitmq.second.username"));
connectionFactory.setPassword(env.getProperty("spring.rabbitmq.second.password"));
return connectionFactory;
}
@Bean(name = "secondRabbitTemplate")
public RabbitTemplate secondRabbitTemplate() {
RabbitTemplate template = new RabbitTemplate(secondConnectionFactory());
return template;
}
}
3. 在需要使用RabbitMQ的地方,注入对应的RabbitTemplate或ConnectionFactory bean即可。例如:
@Autowired
private RabbitTemplate rabbitTemplate;
@Autowired
@Qualifier("secondRabbitTemplate")
private RabbitTemplate secondRabbitTemplate;
使用以上步骤,就可以在Spring Boot应用中配置多个RabbitMQ实例了。
### 回答2:
在Spring Boot中配置多个RabbitMQ会让应用程序变得更加灵活,这意味着应用可以与多个RabbitMQ实例连接,并能够发送和接收消息。
Spring Boot通过在配置文件(application.properties或application.yml)中定义多个RabbitMQ实例来实现多个RabbitMQ的配置。下面是在同一应用程序中配置两个RabbitMQ实例的示例:
在application.yml文件中添加以下内容:
```
spring.rabbitmq.host=server1
spring.rabbitmq.port=5672
spring.rabbitmq.username=user1
spring.rabbitmq.password=password1
spring.rabbitmq.virtual-host=/vhost1
spring.rabbitmq.second.host=server2
spring.rabbitmq.second.port=5672
spring.rabbitmq.second.username=user2
spring.rabbitmq.second.password=password2
spring.rabbitmq.second.virtual-host=/vhost2
```
在上面的示例中,我们定义了两个RabbitMQ实例,一个用于服务器1,另一个用于服务器2。对于每个实例,我们指定了名称,主机名,端口,用户名,密码和虚拟主机。
为了使用以上配置,我们需要通过@Resource注解或@Autowired注解在Java类中定义一个RabbitTemplate bean。同时在需要连接第二个RabbitMQ实例的地方指定使用哪个RabbitMQ实例即可。例如:
```
@SpringBootApplication
public class DemoApplication implements CommandLineRunner {
@Autowired
private RabbitTemplate rabbitTemplate;
@Autowired
@Qualifier("secondRabbitTemplate")
private RabbitTemplate secondRabbitTemplate;
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
@Override
public void run(String... args) throws Exception {
rabbitTemplate.convertAndSend("queue1", "message1");
secondRabbitTemplate.convertAndSend("queue2", "message2");
}
}
```
在上面的代码中,我们注入了两个不同的RabbitTemplate实例,分别用于发送到不同的RabbitMQ实例。我们可以使用这两个实例来发送消息到不同的队列。在这个例子中,我们把“message1”发送到“queue1”,把“message2” 发送到“queue2”。
总之,配置多个RabbitMQ实例可以让Spring Boot应用程序与多个RabbitMQ连接,并实现更复杂的异构应用程序。我们只需要简单地在配置文件中添加多个实例,并通过Java类中的@Resource或@Autowired注解即可使用。
### 回答3:
Spring Boot 是一种流行的 Java 框架,用于快速构建基于 Spring 框架的应用程序。RabbitMQ 是一个流行的开源消息队列,常用于构建分布式系统。Spring Boot 提供了对 RabbitMQ 的支持,并允许用户配置多个 RabbitMQ。
配置多个 RabbitMQ,可以通过在 application.properties 或 application.yml 文件中添加多个 RabbitMQ 的配置来实现。如下所示:
application.yml:
```
spring:
rabbitmq:
1:
host: localhost
port: 5672
username: guest
password: guest
virtual-host: /
2:
host: localhost
port: 5673
username: guest
password: guest
virtual-host: /
...
```
application.properties:
```
spring.rabbitmq.1.host=localhost
spring.rabbitmq.1.port=5672
spring.rabbitmq.1.username=guest
spring.rabbitmq.1.password=guest
spring.rabbitmq.1.virtual-host=/
spring.rabbitmq.2.host=localhost
spring.rabbitmq.2.port=5673
spring.rabbitmq.2.username=guest
spring.rabbitmq.2.password=guest
spring.rabbitmq.2.virtual-host=/
...
```
其中,`1` 和 `2` 表示 RabbitMQ 的标识符。我们可以根据需要添加更多的标识符。在我们的应用程序中,我们可以使用以下方式注入多个RabbitMQ:
```
@Bean
@Primary
public ConnectionFactory connectionFactory1() {
CachingConnectionFactory connectionFactory =
new CachingConnectionFactory("localhost", 5672);
connectionFactory.setUsername("guest");
connectionFactory.setPassword("guest");
return connectionFactory;
}
@Bean
public RabbitTemplate rabbitTemplate1(ConnectionFactory connectionFactory1) {
return new RabbitTemplate(connectionFactory1);
}
@Bean
public SimpleMessageListenerContainer messageListenerContainer1(
ConnectionFactory connectionFactory1) {
SimpleMessageListenerContainer container =
new SimpleMessageListenerContainer(connectionFactory1);
container.setQueueNames("queue1");
container.setDefaultRequeueRejected(false);
container.setMessageListener(messageListenerAdapter1());
return container;
}
@Bean
public MessageListenerAdapter messageListenerAdapter1() {
return new MessageListenerAdapter(new MyMessageListener());
}
@Bean
public ConnectionFactory connectionFactory2() {
CachingConnectionFactory connectionFactory =
new CachingConnectionFactory("localhost", 5673);
connectionFactory.setUsername("guest");
connectionFactory.setPassword("guest");
return connectionFactory;
}
@Bean
public RabbitTemplate rabbitTemplate2(ConnectionFactory connectionFactory2) {
return new RabbitTemplate(connectionFactory2);
}
@Bean
public SimpleMessageListenerContainer messageListenerContainer2(
ConnectionFactory connectionFactory2) {
SimpleMessageListenerContainer container =
new SimpleMessageListenerContainer(connectionFactory2);
container.setQueueNames("queue2");
container.setDefaultRequeueRejected(false);
container.setMessageListener(messageListenerAdapter2());
return container;
}
@Bean
public MessageListenerAdapter messageListenerAdapter2() {
return new MessageListenerAdapter(new MyMessageListener());
}
```
这样,在我们的应用程序中就可以使用`rabbitTemplate1`和`rabbitTemplate2`来发送消息,使用 `messageListenerContainer1`和`messageListenerContainer2`监听队列消息。
总结:
在 Spring Boot 配置多个 RabbitMQ 的过程中,我们需要在 application.properties 或 application.yml 中添加多个配置,注入多个 ConnectionFactory,RabbitTemplate 和 SimpleMessageListenerContainer 来进行发送和监听消息。同时,我们还需要根据实际情况调整配置,确保消息传递的正确性和可靠性。
阅读全文
相关推荐
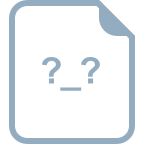
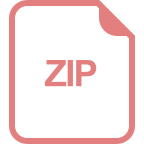

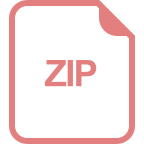

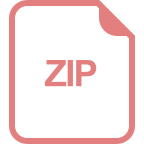
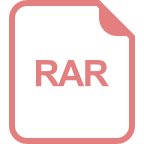
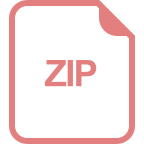
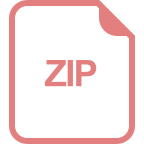
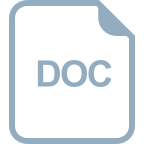
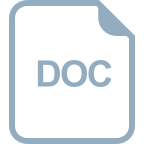
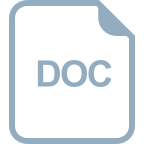
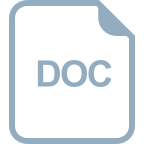
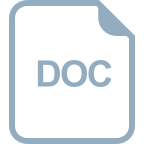
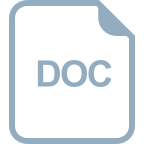
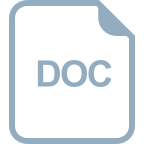
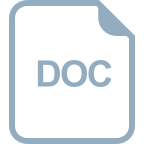
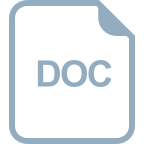