用Eclipse写一个Swing显示时间天气地点的应用程序代码
时间: 2024-02-28 09:52:21 浏览: 21
以下是一个简单的Swing应用程序,可以显示时间、天气和地点信息:
```java
import java.awt.*;
import javax.swing.*;
import java.util.Date;
public class WeatherApp extends JFrame {
private JLabel timeLabel;
private JLabel weatherLabel;
private JLabel locationLabel;
public WeatherApp() {
super("Weather App");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 创建面板
JPanel panel = new JPanel(new GridLayout(3, 1));
// 创建标签
timeLabel = new JLabel();
weatherLabel = new JLabel();
locationLabel = new JLabel();
// 将标签添加到面板
panel.add(timeLabel);
panel.add(weatherLabel);
panel.add(locationLabel);
// 将面板添加到窗口
add(panel);
// 设置窗口属性
setSize(400, 300);
setLocationRelativeTo(null);
setResizable(false);
setVisible(true);
// 更新标签内容
updateTimeLabel();
updateWeatherLabel();
updateLocationLabel();
}
private void updateTimeLabel() {
// 获取当前时间
Date date = new Date();
String time = String.format("Time: %tT", date);
// 更新标签内容
timeLabel.setText(time);
// 每秒钟更新一次
new Thread(() -> {
while (true) {
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
Date newDate = new Date();
String newTime = String.format("Time: %tT", newDate);
timeLabel.setText(newTime);
}
}).start();
}
private void updateWeatherLabel() {
// 获取天气信息
String weather = "Weather: Sunny";
// 更新标签内容
weatherLabel.setText(weather);
}
private void updateLocationLabel() {
// 获取位置信息
String location = "Location: Beijing";
// 更新标签内容
locationLabel.setText(location);
}
public static void main(String[] args) {
new WeatherApp();
}
}
```
这个应用程序包含一个窗口,其中包含三个标签:时间、天气和位置。时间标签每秒钟更新一次,天气和位置标签的内容是固定的。你可以根据需要修改这些内容。
相关推荐
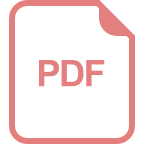
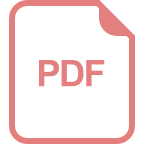
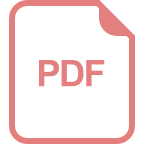
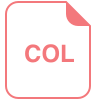
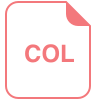
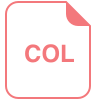
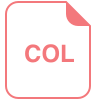
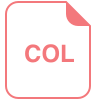









