有个列表['hello', 'world', 'python'],如何把列表里面的字符串联起来,得到字符串 “hello_world_python”? 输入样例1: 在这里给出一组输入。例如: ['hello', 'world', 'python'] 输出样例1: 在这里给出相应的输出。例如: hello_world_python 输入样例2: 在这里给出一组输入。例如: ['hi', 'python', 'man', 'world', '!'] 输出样例2: 在这里给出相应的输出。例如: hi_python_man_world_!
时间: 2023-12-04 08:06:28 浏览: 67
可以使用下划线(_)来连接列表中的字符串,代码如下:
```python
lst = ['hello', 'world', 'python']
result = '_'.join(lst)
print(result)
```
输出结果为:
```
hello_world_python
```
同样的,如果输入是`['hi', 'python', 'man', 'world', '!']`,则输出结果为`hi_python_man_world_!`。
相关问题
有个列表[hello,'world,python'],如何把列表里面的字符串联起来,得到字符串“hello world _python"?
可以使用字符串的 join 方法,代码如下:
```
lst = ['hello', 'world', 'python']
result = ' '.join(lst).replace('_', ' ')
print(result)
```
输出结果为:
```
hello world python
```
有个列表['hello', 'world', 'python'],如何把列表里面的字符串联起来,得到字符串 “hello_world_python”?
### 回答1:
可以使用字符串的join方法,代码如下:
```
lst = ['hello', 'world', 'python']
result = '_'.join(lst)
print(result)
```
输出结果为:
```
hello_world_python
```
### 回答2:
要把列表里面的字符串联起来,有很多种方法,这里介绍两种常见的方法。
第一种方法是使用循环遍历列表,依次拼接字符串。可以定义一个空字符串变量,然后通过 for 循环遍历列表中的每一个字符串,将其与空字符串变量拼接起来。具体代码如下:
```python
words = ['hello', 'world', 'python']
result = ''
for word in words:
result += word + '_'
result = result[:-1]
print(result)
```
这里使用了一个切片操作 `result[:-1]`,它是为了去掉最后一个不需要的下划线。输出结果为:
```
hello_world_python
```
第二种方法是使用字符串的 `join()` 方法,它可以将列表中的多个字符串使用指定的分隔符连接成一个字符串。具体代码如下:
```python
words = ['hello', 'world', 'python']
result = '_'.join(words)
print(result)
```
这里使用了字符串的 `join()` 方法,将列表中的每个字符串用下划线连接起来。输出结果与上面的方法相同:
```
hello_world_python
```
这两个方法都可以将列表中的多个字符串联起来,得到字符串 “hello_world_python”。其中第二种方法更加简洁,使用起来更加方便。
### 回答3:
可以使用Python提供的join()函数,将列表里面的字符串联起来。这个函数的使用方法是str.join(iterable),其中str是将要插入到每个元素之间的字符串,iterable是要连接的元素序列。在这个问题中,我们要得到字符串“hello_world_python”,所以str应该是下划线'_',iterable就是这里的列表['hello', 'world', 'python']。代码如下:
```
lst = ['hello', 'world', 'python']
sep = '_'
result = sep.join(lst)
print(result) # 'hello_world_python'
```
在上面的代码中,我们先定义了一个列表lst和一个字符串sep,它们分别是要连接的元素序列和连接元素之间的字符串。然后,我们使用join()函数将lst里面的元素使用sep连接起来并返回结果给变量result,最后打印result的值。运行上述代码将会输出“hello_world_python”,正是我们想要得到的字符串。
阅读全文
相关推荐


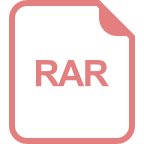

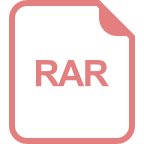
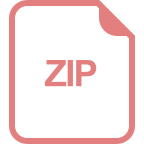
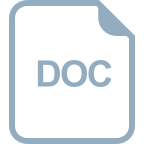
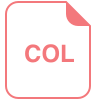




