<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>leon</title> <style> * { margin: 0; padding: 0; } form { width: 500px; padding: 20px; border: 10px solid pink; border-radius: 15px; margin: 50px auto; display: flex; flex-direction: column; } form > label { height: 50px; position: relative; } form > label > span { position: absolute; left: 20px; bottom: 5px; color: red; display: none; } form > label > span.active { display: block; } </style> </head> <body> <form action=""> <label for=""> 用户名 : <input class="username" type="text"> <span>用户名必须字母开头6-12位, 请重新填写 ^^</span> </label> <label for=""> 密 码 : <input class="password" type="text"> <span>密码必须6-12位, 请重新填写 ^^</span> </label> <label for=""> 手机号 : <input class="phone" type="text"> <span>手机号不符合规则, 请重新填写 ^^</span> </label> <label for=""> 昵 称 : <input class="nickname" type="text"> <span>昵称必须3~5位中文, 请重新填写 ^^</span> </label> <button>提交</button> </form> </body> </html> <script src="./index.js" type="module"></script>请编写js代码实现功能,并满足在输入时验证不正确显示active样式
时间: 2023-08-14 18:37:44 浏览: 133
好的,您可以尝试使用以下 JavaScript 代码实现您的功能需求:
```js
const form = document.querySelector('form');
const usernameInput = document.querySelector('.username');
const passwordInput = document.querySelector('.password');
const phoneInput = document.querySelector('.phone');
const nicknameInput = document.querySelector('.nickname');
const usernameSpan = document.querySelector('.username + span');
const passwordSpan = document.querySelector('.password + span');
const phoneSpan = document.querySelector('.phone + span');
const nicknameSpan = document.querySelector('.nickname + span');
function checkUsername() {
const username = usernameInput.value.trim();
const regex = /^[a-zA-Z]\w{5,11}$/;
if (!regex.test(username)) {
usernameSpan.classList.add('active');
return false;
} else {
usernameSpan.classList.remove('active');
return true;
}
}
function checkPassword() {
const password = passwordInput.value.trim();
if (password.length < 6 || password.length > 12) {
passwordSpan.classList.add('active');
return false;
} else {
passwordSpan.classList.remove('active');
return true;
}
}
function checkPhone() {
const phone = phoneInput.value.trim();
const regex = /^1[3-9]\d{9}$/;
if (!regex.test(phone)) {
phoneSpan.classList.add('active');
return false;
} else {
phoneSpan.classList.remove('active');
return true;
}
}
function checkNickname() {
const nickname = nicknameInput.value.trim();
const regex = /^[\u4e00-\u9fa5]{3,5}$/;
if (!regex.test(nickname)) {
nicknameSpan.classList.add('active');
return false;
} else {
nicknameSpan.classList.remove('active');
return true;
}
}
function checkForm() {
const isUsernameValid = checkUsername();
const isPasswordValid = checkPassword();
const isPhoneValid = checkPhone();
const isNicknameValid = checkNickname();
return isUsernameValid && isPasswordValid && isPhoneValid && isNicknameValid;
}
form.addEventListener('submit', (event) => {
event.preventDefault();
if (checkForm()) {
alert('恭喜,验证通过!');
} else {
alert('很抱歉,验证失败,请检查输入!');
}
});
usernameInput.addEventListener('input', checkUsername);
passwordInput.addEventListener('input', checkPassword);
phoneInput.addEventListener('input', checkPhone);
nicknameInput.addEventListener('input', checkNickname);
```
这段代码主要做了以下几个事情:
1. 通过 `querySelector` 方法获取表单元素和相关的输入框和提示文本元素;
2. 分别编写了 4 个验证函数,用于验证用户名、密码、手机号和昵称的格式是否符合要求,并在验证失败时添加 `active` 类名以显示提示文本;
3. 编写了一个 `checkForm` 函数,用于检查整个表单是否通过验证;
4. 在表单提交事件中,阻止默认行为,调用 `checkForm` 函数检查表单是否通过验证,并根据结果显示相应的提示信息;
5. 在输入框的输入事件中,调用相应的验证函数进行实时验证。
通过这样的代码实现,用户在输入时会实时得到验证的反馈,如果输入不合法,则会显示相应的提示文本。如果用户提交表单时,表单中有任何一个输入框不合法,则会提示用户重新检查输入。如果全部通过验证,则会提示用户验证通过。同时,不正确的输入会添加 `active` 类名来显示提示文本,让用户更加直观地了解错误原因。
阅读全文
相关推荐
















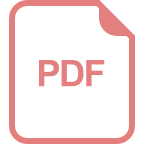

