读取电脑上的文件文本,写出python代码
时间: 2023-07-18 17:13:56 浏览: 52
可以使用Python内置的open函数来读取电脑上的文件文本,具体步骤如下:
1. 使用open函数打开文件,指定文件路径和打开模式,例如:
```python
file_path = 'path/to/your/file.txt'
with open(file_path, 'r', encoding='utf-8') as f:
text = f.read()
```
其中,'r'表示读取模式,encoding参数指定文件编码。
2. 读取文件内容,可以使用read()方法将整个文件一次性读入内存,或者使用readline()方法逐行读取,例如:
```python
# 一次性读入整个文件
file_path = 'path/to/your/file.txt'
with open(file_path, 'r', encoding='utf-8') as f:
text = f.read()
# 逐行读取文件
file_path = 'path/to/your/file.txt'
with open(file_path, 'r', encoding='utf-8') as f:
for line in f:
print(line)
```
注意:在使用open函数读取文件时,需要使用with语句来确保文件被正确关闭,避免出现文件泄漏等问题。
相关问题
如何读取句子在前,标签在后的TXT文本文件,写出Python代码
假设文本文件中每一行的格式为:句子 TAB 标签,可以使用 Python 中的 split() 方法将句子和标签分开,然后将它们存储到不同的列表中。以下是读取文本文件的 Python 代码示例:
```python
sentences = []
labels = []
with open('text_file.txt', 'r') as f:
for line in f:
line = line.strip() # 删除行末的换行符
sentence, label = line.split('\t')
sentences.append(sentence)
labels.append(label)
```
这样,你就可以得到一个包含所有句子的列表 `sentences` 和一个包含所有标签的列表 `labels`。接下来,你可以根据需要对它们进行进一步的处理。
用python写出弯曲文本矫正代码
我可以回答这个问题。弯曲文本矫正是指将弯曲的文本图像进行矫正,使其变为水平或竖直方向。这个问题可以通过使用OpenCV等图像处理库和算法来解决。具体步骤包括:读取图像、提取文本区域、将文本区域二值化、计算文本区域的倾斜角度、旋转文本区域、输出矫正后的图像。如果你需要更具体的实现方法,可以参考相关的Python代码库和教程。
相关推荐
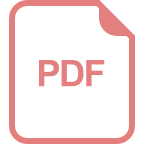
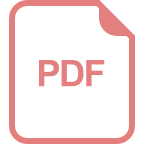
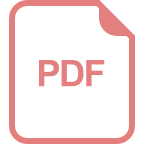












