coco数据集转换成yolo数据集
时间: 2023-10-20 15:06:42 浏览: 126
将COCO数据集转换为YOLO数据集可以通过以下步骤完成:
1. 下载COCO数据集并解压缩。
2. 安装COCO API和OpenCV。
3. 创建一个新的文件夹,用于存储转换后的YOLO数据集。
4. 读取COCO中的图像和注释。
5. 将每个图像转换为YOLO格式(.txt文件)。
6. 将所有的YOLO格式文件和图像放入新的文件夹中。
以下是一个将COCO数据集转换为YOLO数据集的Python代码示例:
```python
import os
import json
import cv2
# COCO类别对应的YOLO类别
class_id = {
"person": 0,
"bicycle": 1,
"car": 2,
"motorcycle": 3,
"airplane": 4,
"bus": 5,
"train": 6,
"truck": 7,
"boat": 8,
"traffic light": 9,
"fire hydrant": 10,
"stop sign": 11,
"parking meter": 12,
"bench": 13,
"bird": 14,
"cat": 15,
"dog": 16,
"horse": 17,
"sheep": 18,
"cow": 19,
"elephant": 20,
"bear": 21,
"zebra": 22,
"giraffe": 23,
"backpack": 24,
"umbrella": 25,
"handbag": 26,
"tie": 27,
"suitcase": 28,
"frisbee": 29,
"skis": 30,
"snowboard": 31,
"sports ball": 32,
"kite": 33,
"baseball bat": 34,
"baseball glove": 35,
"skateboard": 36,
"surfboard": 37,
"tennis racket": 38,
"bottle": 39,
"wine glass": 40,
"cup": 41,
"fork": 42,
"knife": 43,
"spoon": 44,
"bowl": 45,
"banana": 46,
"apple": 47,
"sandwich": 48,
"orange": 49,
"broccoli": 50,
"carrot": 51,
"hot dog": 52,
"pizza": 53,
"donut": 54,
"cake": 55,
"chair": 56,
"couch": 57,
"potted plant": 58,
"bed": 59,
"dining table": 60,
"toilet": 61,
"tv": 62,
"laptop": 63,
"mouse": 64,
"remote": 65,
"keyboard": 66,
"cell phone": 67,
"microwave": 68,
"oven": 69,
"toaster": 70,
"sink": 71,
"refrigerator": 72,
"book": 73,
"clock": 74,
"vase": 75,
"scissors": 76,
"teddy bear": 77,
"hair drier": 78,
"toothbrush": 79
}
# 将COCO注释转换为YOLO格式
def convert_annotation(image_id, coco_annotation, file):
for annotation in coco_annotation:
x, y, w, h = annotation['bbox']
x_center = x + w / 2
y_center = y + h / 2
x_center /= width
y_center /= height
w /= width
h /= height
class_name = coco_classes[annotation["category_id"]]
class_num = class_id[class_name]
file.write("%s %s %s %s %s\n" % (class_num, x_center, y_center, w, h))
# COCO数据集文件夹路径
coco_folder = "/path/to/coco/folder"
# 用于存储YOLO数据集的文件夹路径
yolo_folder = "/path/to/yolo/folder"
# COCO数据集注释文件
coco_annotation_file = os.path.join(coco_folder, "annotations/instances_val2017.json")
# COCO数据集图像文件夹
coco_image_folder = os.path.join(coco_folder, "val2017")
# 创建一个新的文件夹,用于存储转换后的YOLO数据集
if not os.path.exists(yolo_folder):
os.makedirs(yolo_folder)
# 读取COCO注释文件
with open(coco_annotation_file) as f:
coco_data = json.load(f)
# COCO类别列表
coco_classes = []
for category in coco_data['categories']:
coco_classes.append(category['name'])
# 遍历COCO数据集中的每个图像
for image_data in coco_data['images']:
# 获取图像文件名和路径
image_name = image_data['file_name']
image_path = os.path.join(coco_image_folder, image_name)
# 读取图像
image = cv2.imread(image_path)
height, width, channels = image.shape
# 创建YOLO格式的注释文件
yolo_annotation_file = os.path.join(yolo_folder, os.path.splitext(image_name)[0] + ".txt")
with open(yolo_annotation_file, "w") as f:
# 获取图像中的每个注释
for annotation in coco_data['annotations']:
# 如果注释属于当前图像,则将其转换为YOLO格式
if annotation['image_id'] == image_data['id']:
convert_annotation(image_data['id'], [annotation], f)
# 将图像复制到YOLO数据集文件夹中
yolo_image_path = os.path.join(yolo_folder, image_name)
cv2.imwrite(yolo_image_path, image)
```
在运行完上述代码后,YOLO数据集文件夹中应该包括所有转换后的图像和注释文件。
阅读全文
相关推荐
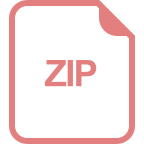
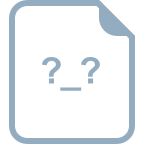



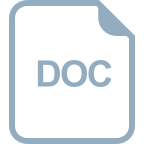




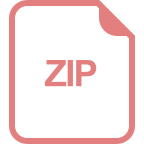
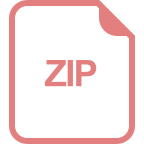
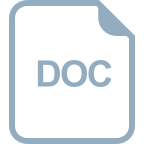



