#include <iostream> #include<stdlib.h> using namespace std; class date_class { private: int day, month, year; public: void setdate(int d,int y,int m){ cout<<"please enter day"; cin>>d; day=d; cout<<"please enter month"; cin>>m; month=m; cout<<"please enter year"; cin>>y; year=y; } int getday(); int getmonth(); int getyear(); void displaydate(); }; date_class today; int main() { int m; today.setdate(); today.displaydate(); m = today.getmonth(); // m = today.month; -- causes an error because month is private cout << "The month in this date is " << m << endl; system("pause"); } int date_class::getday() { return day; } int date_class::getmonth() { return month; } int date_class::getyear() { return year; } void date_class::displaydate() { cout << day << "/" << month << "/" << year << endl; } 怎么改进
时间: 2024-01-29 14:02:24 浏览: 140
这段代码存在一些问题,主要是在 setdate 函数中将传入的参数忽略了,而是通过 cin 获取输入。另外,类中的成员变量应该设置为私有,通过公有的成员函数来获取和修改。
以下是一些可能的改进:
1. 修改 setdate 函数,使用传入的参数来设置日期:
```
void setdate(int d, int m, int y) {
day = d;
month = m;
year = y;
}
```
2. 将成员变量设为私有,并添加公有的成员函数来获取和修改日期:
```
class date_class {
private:
int day, month, year;
public:
void setdate(int d, int m, int y) {
day = d;
month = m;
year = y;
}
int getday() const { return day; }
int getmonth() const { return month; }
int getyear() const { return year; }
void displaydate() const {
cout << day << "/" << month << "/" << year << endl;
}
};
```
3. 在 main 函数中调用 setdate 函数时,传入实际的日期参数:
```
int main() {
int d, m, y;
cout << "Enter day: ";
cin >> d;
cout << "Enter month: ";
cin >> m;
cout << "Enter year: ";
cin >> y;
today.setdate(d, m, y);
today.displaydate();
int m = today.getmonth();
cout << "The month in this date is " << m << endl;
system("pause");
}
```
这样就可以通过传入参数来设置日期,同时也可以通过公有成员函数来获取和修改日期。
阅读全文
相关推荐










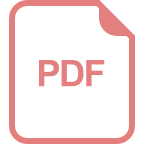


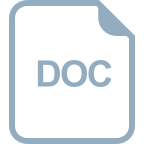



