opencv/c++ 检测管道形变的代码
时间: 2024-02-07 09:03:40 浏览: 144
以下是使用OpenCV和C++实现的检测管道形变的代码示例:
```c++
#include <opencv2/core.hpp>
#include <opencv2/highgui.hpp>
#include <opencv2/imgproc.hpp>
using namespace cv;
int main()
{
// 读取原始图像
Mat src = imread("pipe.jpg");
// 将图像转换为灰度图像
Mat gray;
cvtColor(src, gray, COLOR_BGR2GRAY);
// 对图像进行二值化处理
Mat binary;
threshold(gray, binary, 0, 255, THRESH_BINARY | THRESH_OTSU);
// 进行边缘检测
Mat edges;
Canny(binary, edges, 50, 200, 3);
// 进行霍夫直线变换
std::vector<Vec2f> lines;
HoughLines(edges, lines, 1, CV_PI / 180, 100, 0, 0);
// 绘制直线
Mat result = src.clone();
for (size_t i = 0; i < lines.size(); i++)
{
float rho = lines[i][0], theta = lines[i][1];
Point pt1, pt2;
double a = cos(theta), b = sin(theta);
double x0 = a * rho, y0 = b * rho;
pt1.x = cvRound(x0 + 1000 * (-b));
pt1.y = cvRound(y0 + 1000 * (a));
pt2.x = cvRound(x0 - 1000 * (-b));
pt2.y = cvRound(y0 - 1000 * (a));
line(result, pt1, pt2, Scalar(0, 0, 255), 2, LINE_AA);
}
// 显示结果
imshow("Result", result);
waitKey(0);
return 0;
}
```
以上代码会读取一张名为`pipe.jpg`的图像,并进行二值化、边缘检测和霍夫直线变换,最后将检测出的直线绘制在原图上并显示出来。你也可以根据自己的需要对代码进行修改。
阅读全文
相关推荐
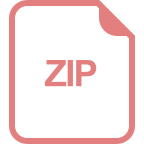
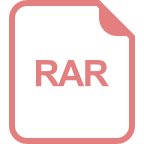
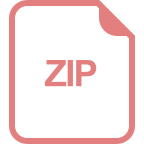
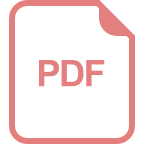
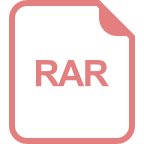
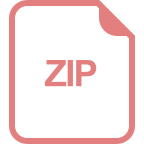
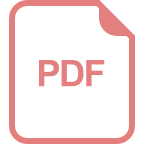
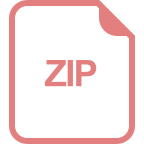
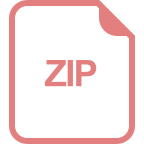
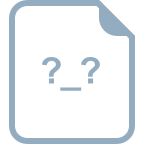
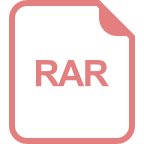
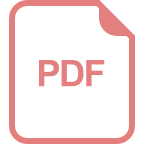
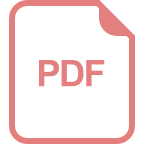
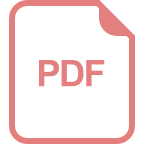
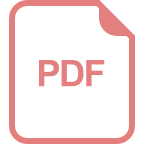
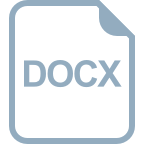