采用面向对象编程思想,编写一个超市购物的程序。
时间: 2023-06-19 13:02:57 浏览: 104
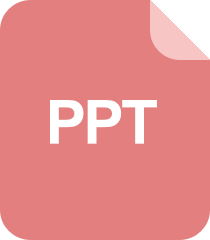
面向对象编程思想-alex.ppt
好的,这是一个简单的超市购物程序的代码示例:
```python
class Product:
def __init__(self, name, price):
self.name = name
self.price = price
class Cart:
def __init__(self):
self.items = []
def add_item(self, product, quantity):
self.items.append((product, quantity))
def remove_item(self, item):
self.items.remove(item)
def calculate_total(self):
total = 0
for item in self.items:
product, quantity = item
total += product.price * quantity
return total
class Supermarket:
def __init__(self):
self.products = [Product("Apple", 2), Product("Banana", 3), Product("Pear", 1.5), Product("Milk", 4)]
def display_products(self):
print("Products available:")
for i, product in enumerate(self.products):
print(f"{i + 1}. {product.name} - ${product.price}")
def checkout(self, cart):
total = cart.calculate_total()
print(f"Total: ${total:.2f}")
while True:
payment = float(input("Enter payment amount: $"))
if payment < total:
print("Payment amount is less than total.")
else:
break
change = payment - total
print(f"Change: ${change:.2f}")
print("Thank you for shopping with us!")
# Example usage
market = Supermarket()
market.display_products()
cart = Cart()
cart.add_item(market.products[0], 2)
cart.add_item(market.products[1], 1)
market.checkout(cart)
```
此程序使用 `Product` 类来表示每个商品的名称和价格。 `Cart` 类存储购物车中的商品和数量,并提供计算总价的方法。 `Supermarket` 类管理可用商品,并提供结帐功能。
在主程序中,我们首先创建了一个超市对象,然后显示了可用商品。然后我们创建了一个购物车对象,并添加了一些商品。最后,我们使用超市对象进行结帐,并要求用户输入付款金额,直到输入的金额大于或等于总价。程序输出找零,并显示感谢消息。
阅读全文
相关推荐
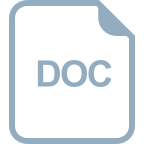
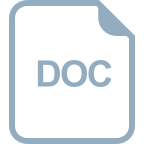
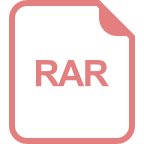
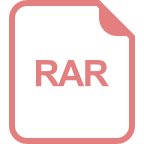
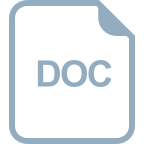
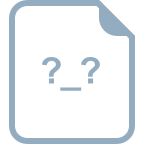
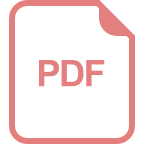
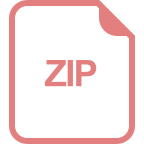
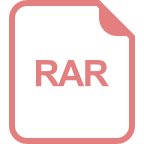
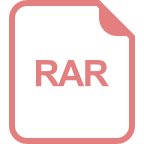
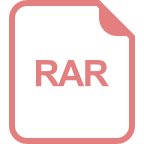
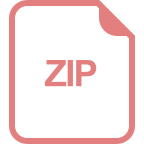
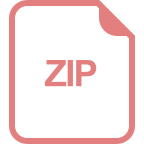
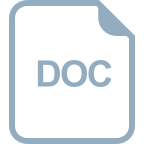
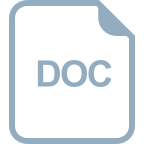
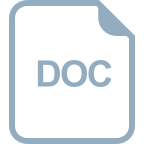
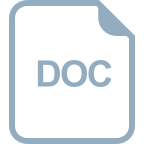
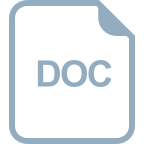