private void alarmTimer_Tick(object sender, EventArgs e) { MessageBox.Show("天选之子,起床了迎接朝阳!", "闹钟提醒", MessageBoxButtons.OK, MessageBoxIcon.Information); alarmTimer.Stop(); // 获取当前时间 DateTime currentTime = DateTime.Now; // 获取设定的闹钟时间 DateTime alarmTime = alarmTimePicker.Value; // 如果当前时间和设定的闹钟时间一致,则播放音乐 if (currentTime.Hour == alarmTime.Hour && currentTime.Minute == alarmTime.Minute && currentTime.Second == alarmTime.Second) { System.Media.SoundPlayer player = new System.Media.SoundPlayer(); player.SoundLocation = "C:\\Users\\HP\\Music\\SWIN-只因你太美.mp3"; player.Play(); } }这样就行了吧
时间: 2024-02-15 11:28:50 浏览: 21
这段代码看起来是一个在 Windows 窗体应用程序中实现闹钟功能的代码。当 `alarmTimer` 定时器触发时,会弹出一个消息框提示用户起床,并且获取当前时间和设定的闹钟时间进行比较,如果相同则播放音乐。但是,这段代码中只是简单地使用了 `MessageBox` 和 `System.Media.SoundPlayer` 进行提示和音乐播放,并没有考虑到一些问题,例如:
1. 当用户关闭了消息框后,闹钟是否会继续响起?
2. 如果用户在闹钟响起时关闭了应用程序,闹钟是否会停止?
3. 是否考虑到用户设置多个闹钟的情况?
因此,如果您想要实现一个更完整、更稳定的闹钟功能,可以考虑使用第三方库或者更加完善的代码实现。
相关问题
private void timer2_Tick(object sender, EventArgs e)
这段代码是一个事件处理函数,当名为"timer2"的计时器组件触发Tick事件时会被调用。Tick事件是计时器组件的一个重要事件,它在计时器周期性地达到指定时间间隔时触发,可以用来实现一些周期性的操作。在这个事件处理函数中可以编写一些代码来响应Tick事件,例如更新界面元素、执行一些操作或者控制程序的运行状态等等。由于这个函数的具体实现是根据具体需求而定的,所以无法确定这个函数的具体作用。需要查看完整的代码才能确定这个函数实现的功能。
private void alarmTimer_Tick(object sender, EventArgs e) { MessageBox.Show("天选之子,起床了迎接朝阳!", "闹钟提醒", MessageBoxButtons.OK, MessageBoxIcon.Information); alarmTimer.Stop(); // 获取当前时间 DateTime currentTime = DateTime.Now; // 获取设定的闹钟时间 DateTime alarmTime = alarmTimePicker.Value; // 如果当前时间和设定的闹钟时间一致,则播放音乐 if (currentTime.Hour == alarmTime.Hour && currentTime.Minute == alarmTime.Minute && currentTime.Second == alarmTime.Second) { System.Media.SoundPlayer player = new System.Media.SoundPlayer(); player.SoundLocation = "path_to_sound_file"; player.Play(); } }分析代码
这是一个 C# 的计时器事件处理函数。当计时器触发时,会弹出一个消息框提醒用户起床,并停止计时器计时。
代码中获取了当前时间和设定的闹钟时间,如果它们一致,则会播放音乐。其中,音乐文件的路径应该替换为实际的文件路径。
需要注意的是,代码中并没有定义 alarmTimer,这可能是在其他地方定义的计时器对象。
相关推荐
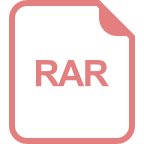













