用java编写一个能够转换华氏温度和摄氏温度的程序。在”摄氏温度“文本域输入一个值并点击按华氏按钮,在华氏文本域中显示相应的华氏温度,反之同理。
时间: 2024-03-15 08:47:49 浏览: 19
好的,以下是Java代码实现:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class TemperatureConverter extends JFrame implements ActionListener {
private JTextField celsiusField;
private JTextField fahrenheitField;
private JButton toFahrenheitButton;
private JButton toCelsiusButton;
public TemperatureConverter() {
super("Temperature Converter");
// 创建组件
JLabel celsiusLabel = new JLabel("摄氏温度:");
celsiusField = new JTextField(10);
JLabel fahrenheitLabel = new JLabel("华氏温度:");
fahrenheitField = new JTextField(10);
fahrenheitField.setEditable(false); // 不可编辑
toFahrenheitButton = new JButton("按华氏");
toCelsiusButton = new JButton("按摄氏");
// 添加组件
JPanel contentPane = new JPanel(new GridLayout(3, 2, 5, 5));
contentPane.add(celsiusLabel);
contentPane.add(celsiusField);
contentPane.add(fahrenheitLabel);
contentPane.add(fahrenheitField);
contentPane.add(toFahrenheitButton);
contentPane.add(toCelsiusButton);
setContentPane(contentPane);
// 注册监听器
toFahrenheitButton.addActionListener(this);
toCelsiusButton.addActionListener(this);
// 设置窗口属性
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
pack();
setVisible(true);
}
// 实现 ActionListener 接口
public void actionPerformed(ActionEvent e) {
if (e.getSource() == toFahrenheitButton) {
// 摄氏度转华氏度
try {
double celsius = Double.parseDouble(celsiusField.getText());
double fahrenheit = celsius * 9 / 5 + 32;
fahrenheitField.setText(String.format("%.1f", fahrenheit));
} catch (NumberFormatException ex) {
JOptionPane.showMessageDialog(this, "请输入合法的数字!", "错误", JOptionPane.ERROR_MESSAGE);
}
} else if (e.getSource() == toCelsiusButton) {
// 华氏度转摄氏度
try {
double fahrenheit = Double.parseDouble(fahrenheitField.getText());
double celsius = (fahrenheit - 32) * 5 / 9;
celsiusField.setText(String.format("%.1f", celsius));
} catch (NumberFormatException ex) {
JOptionPane.showMessageDialog(this, "请输入合法的数字!", "错误", JOptionPane.ERROR_MESSAGE);
}
}
}
public static void main(String[] args) {
new TemperatureConverter();
}
}
```
这个程序使用了 Swing 组件库来创建 GUI 界面,包括文本域、标签和按钮等。当用户点击“按华氏”按钮时,程序会读取摄氏度文本域中的数值,将其转换为华氏度后显示在华氏度文本域中;当用户点击“按摄氏”按钮时,程序会读取华氏度文本域中的数值,将其转换为摄氏度后显示在摄氏度文本域中。如果用户输入的不是数字,则会弹出错误提示框。
相关推荐
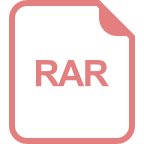
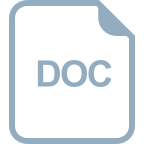














