优化这段代码import cv2 import imutils import numpy as np img = cv2.imread('D:\pycharm\PycharmProjects\pythonProject\p1\p1.jpg', cv2.IMREAD_COLOR) img = cv2.resize(img, (600, 400)) cv2.imshow('Origin image', img) img_gray = cv2.cvtColor(img, cv2.COLOR_RGB2GRAY) img_gray = cv2.bilateralFilter(img_gray, 13, 15, 15) img_edged = cv2.Canny(img_gray, 30, 200) cv2.imshow('edged image', img_edged) img_contours = cv2.findContours(img_edged.copy(), cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE) img_contours = imutils.grab_contours(img_contours) img_contours = sorted(img_contours, key=cv2.contourArea, reverse=True)[:10] screenCnt = None for c in img_contours: peri = cv2.arcLength(c, True) approx = cv2.approxPolyDP(c, 0.018 * peri, True) if len(approx) == 4: screenCnt = approx break if screenCnt is None: detected = 0 print("No contour detected") else: detected = 1 if detected == 1: cv2.drawContours(img, [screenCnt], -1, (0, 0, 255), 3) mask = np.zeros(img_gray.shape, np.uint8) new_image = cv2.drawContours(mask, [screenCnt], 0, 255, -1, ) cv2.imshow('mask_image', new_image) new_image = cv2.bitwise_and(img, img, mask=mask) (x, y) = np.where(mask == 255) (topx, topy) = (np.min(x), np.min(y)) (bottomx, bottomy) = (np.max(x), np.max(y)) cropped = img_gray[topx:bottomx + 1, topy:bottomy + 1] cropped = cv2.resize(cropped, (400, 200)) cv2.imshow('Cropped', cropped) cv2.waitKey(0) cv2.destroyAllWindows()
时间: 2024-04-17 19:23:29 浏览: 39
这段代码主要是对一张图片进行边缘检测,并找出图片中的一个矩形区域进行裁剪。下面是对代码的一些优化建议:
1. 将代码拆分成多个函数,增加代码的可读性和可维护性。
2. 将文件路径字符串改为使用原始字符串(raw string),以避免转义字符带来的问题。
3. 考虑使用命令行参数或配置文件来指定输入文件路径和其他参数,以增加代码的灵活性。
4. 添加错误处理机制,例如检查文件是否存在,处理可能出现的异常情况。
5. 使用`with`语句来管理资源,例如`cv2.VideoCapture`和`cv2.imshow`,以确保资源的正确释放。
6. 使用`argparse`模块来解析命令行参数,以方便指定不同的参数和选项。
7. 使用更具描述性的变量名,以增加代码的可读性。
8. 添加注释来解释代码的功能和逻辑。
9. 考虑使用适当的异常处理机制,例如在无法找到矩形区域时抛出异常或返回错误码。
以上是对代码的一些优化建议,你可以根据实际需求进行调整。
相关问题
优化这段代码import os import cv2 import numpy as np def MediumFilter(img_path='D:\pythonProject2\111.jpg'): img_src=cv2.imread(img_path) img=cv2.resize(src=img_src, dsize=(450, 450)) img=cv2.medianBlur(img, ksize=5) cv2.imshow('img', img) cv2.imshow('img_src', img_src) cv2.waitKey(0) cv2.destroyAllWindows() if __name__ == '__main__': print('Pycharm') MediumFilter()
这段代码可以进行如下优化:
1. 将图片路径作为参数传入函数中,而不是在函数内部指定。这样可以使代码更加灵活,适用于不同的图片路径。
2. 将图片大小的调整和中值滤波分开进行,这样可以更好地控制每个操作的效果。
3. 添加异常处理,以防止程序因为无法打开图片而崩溃。
重构后的代码如下:
```
import os
import cv2
import numpy as np
def medium_filter(img_path):
try:
img_src = cv2.imread(img_path)
img_resized = cv2.resize(src=img_src, dsize=(450, 450))
img_filtered = cv2.medianBlur(img_resized, ksize=5)
cv2.imshow('img_filtered', img_filtered)
cv2.imshow('img_src', img_src)
cv2.waitKey(0)
cv2.destroyAllWindows()
except Exception as e:
print(f"Error: {e}")
if __name__ == '__main__':
img_path = 'D:\pythonProject2\111.jpg'
medium_filter(img_path)
```
这样代码更加简洁、容错性更好、可读性更高。
import numpy as npimport cv2# 读取图像img = cv2.imread('lena.png', 0)# 添加高斯噪声mean = 0var = 0.1sigma = var ** 0.5noise = np.random.normal(mean, sigma, img.shape)noisy_img = img + noise# 定义维纳滤波器函数def wiener_filter(img, psf, K=0.01): # 计算傅里叶变换 img_fft = np.fft.fft2(img) psf_fft = np.fft.fft2(psf) # 计算功率谱 img_power = np.abs(img_fft) ** 2 psf_power = np.abs(psf_fft) ** 2 # 计算信噪比 snr = img_power / (psf_power + K) # 计算滤波器 result_fft = img_fft * snr / psf_fft result = np.fft.ifft2(result_fft) # 返回滤波结果 return np.abs(result)# 定义维纳滤波器的卷积核kernel_size = 3kernel = np.ones((kernel_size, kernel_size)) / kernel_size ** 2# 计算图像的自相关函数acf = cv2.calcHist([img], [0], None, [256], [0, 256])# 计算维纳滤波器的卷积核gamma = 0.1alpha = 0.5beta = 1 - alpha - gammapsf = np.zeros((kernel_size, kernel_size))for i in range(kernel_size): for j in range(kernel_size): i_shift = i - kernel_size // 2 j_shift = j - kernel_size // 2 psf[i, j] = np.exp(-np.pi * ((i_shift ** 2 + j_shift ** 2) / (2 * alpha ** 2))) * np.cos(2 * np.pi * (i_shift + j_shift) / (2 * beta))psf = psf / np.sum(psf)# 对带噪声图像进行维纳滤波filtered_img = wiener_filter(noisy_img, psf)# 显示结果cv2.imshow('Original Image', img)cv2.imshow('Noisy Image', noisy_img)cv2.imshow('Filtered Image', filtered_img)cv2.waitKey(0)cv2.destroyAllWindows()这段代码报错为Traceback (most recent call last): File "<input>", line 1, in <module> File "D:\Pycharm\PyCharm 2020.3.5\plugins\python\helpers\pydev\_pydev_bundle\pydev_umd.py", line 197, in runfile pydev_imports.execfile(filename, global_vars, local_vars) # execute the script File "D:\Pycharm\PyCharm 2020.3.5\plugins\python\helpers\pydev\_pydev_imps\_pydev_execfile.py", line 18, in execfile exec(compile(contents+"\n", file, 'exec'), glob, loc) File "E:/Python_project/class_/weinalvboqi.py", line 54, in <module> filtered_img = wiener_filter(noisy_img, psf) File "E:/Python_project/class_/weinalvboqi.py", line 25, in wiener_filter snr = img_power / (psf_power + K) ValueError: operands could not be broadcast together with shapes (1024,2800) (3,3)什么意思,如何修改
这个错误的意思是图像的噪声卷积核和维纳滤波器的卷积核形状不匹配,导致无法进行计算。可以尝试将维纳滤波器的卷积核的大小调整为与图像的噪声卷积核相同的大小,或者将图像的噪声卷积核的大小调整为与维纳滤波器的卷积核相同的大小。同时,还需要注意在计算傅里叶变换和功率谱时,需要对卷积核进行中心化处理,即将卷积核的中心移动到图像的中心位置。
相关推荐














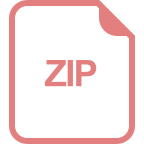
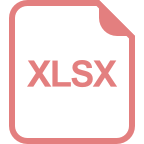
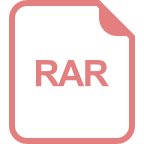