利用html,css和js制作简单日历
时间: 2023-10-03 09:07:01 浏览: 124
好的,以下是一个简单的日历制作过程:
1. HTML 结构
首先,我们需要定义一个 HTML 结构来构建日历。可以使用 `<table>` 标签来实现。代码如下:
```html
<table>
<thead>
<tr>
<th colspan="7" class="month"></th>
</tr>
<tr>
<th>日</th>
<th>一</th>
<th>二</th>
<th>三</th>
<th>四</th>
<th>五</th>
<th>六</th>
</tr>
</thead>
<tbody class="days"></tbody>
</table>
```
2. CSS 样式
接着,我们需要添加一些 CSS 样式来美化日历。可以使用 CSS3 的 flexbox 布局,让日历表格更加美观。代码如下:
```css
table {
border-collapse: collapse;
width: 100%;
}
th {
padding: 10px;
text-align: center;
}
td {
padding: 10px;
text-align: center;
border: 1px solid #ccc;
}
.month {
font-size: 20px;
font-weight: bold;
}
.days {
display: flex;
flex-wrap: wrap;
justify-content: space-between;
}
```
3. JavaScript 逻辑
最后,我们需要使用 JavaScript 来动态生成日历内容。可以使用 Date() 对象来获取当前日期和月份,然后根据当前月份生成当月的日期。代码如下:
```javascript
// 获取当前日期和月份
const date = new Date();
let year = date.getFullYear();
let month = date.getMonth() + 1;
// 设置月份
const monthEl = document.querySelector('.month');
monthEl.innerText = `${year}年${month}月`;
// 获取当月天数
const days = new Date(year, month, 0).getDate();
// 生成当月日期
const daysEl = document.querySelector('.days');
let html = '';
for (let i = 1; i <= days; i++) {
html += `<td>${i}</td>`;
}
daysEl.innerHTML = html;
```
以上就是制作简单日历的过程,你可以根据自己的需求进行样式和功能的更改。
阅读全文
相关推荐
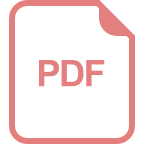
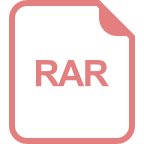
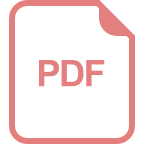
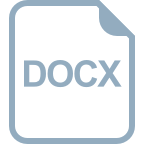
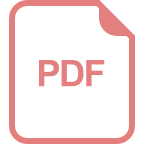
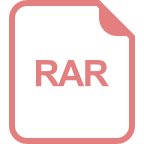
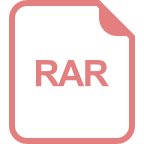
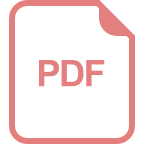
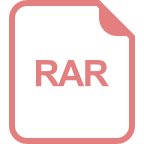
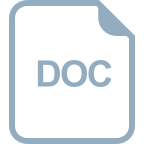
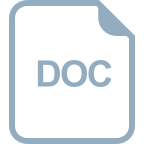
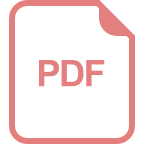
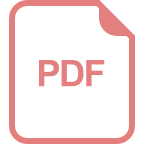
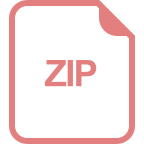
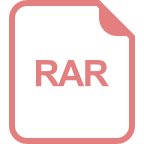
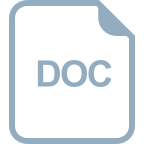