请使用unity 脚本实现一个A*寻路算法
时间: 2023-03-21 11:03:27 浏览: 247
好的,我会尝试回答你的问题。
首先,要使用Unity脚本实现A*寻路算法,需要在Unity中创建一个脚本,并将其添加到场景中的游戏对象上。
以下是实现A*寻路算法的Unity脚本示例代码:
```csharp
using UnityEngine;
using System.Collections;
using System.Collections.Generic;
public class AStarPathfinding : MonoBehaviour {
public Transform seeker, target; //起点和终点
Grid grid; //寻路所需的网格
void Awake() {
grid = GetComponent<Grid>();
}
void Update() {
FindPath(seeker.position, target.position);
}
void FindPath(Vector3 startPos, Vector3 targetPos) {
Node startNode = grid.NodeFromWorldPoint(startPos);
Node targetNode = grid.NodeFromWorldPoint(targetPos);
List<Node> openSet = new List<Node>();
HashSet<Node> closedSet = new HashSet<Node>();
openSet.Add(startNode);
while (openSet.Count > 0) {
Node currentNode = openSet[0];
for (int i = 1; i < openSet.Count; i++) {
if (openSet[i].fCost < currentNode.fCost || (openSet[i].fCost == currentNode.fCost && openSet[i].hCost < currentNode.hCost)) {
currentNode = openSet[i];
}
}
openSet.Remove(currentNode);
closedSet.Add(currentNode);
if (currentNode == targetNode) {
RetracePath(startNode, targetNode);
return;
}
foreach (Node neighbour in grid.GetNeighbours(currentNode)) {
if (!neighbour.walkable || closedSet.Contains(neighbour)) {
continue;
}
int newMovementCostToNeighbour = currentNode.gCost + GetDistance(currentNode, neighbour);
if (newMovementCostToNeighbour < neighbour.gCost || !openSet.Contains(neighbour)) {
neighbour.gCost = newMovementCostToNeighbour;
neighbour.hCost = GetDistance(neighbour, targetNode);
neighbour.parent = currentNode;
if (!openSet.Contains(neighbour)) {
openSet.Add(neighbour);
}
}
}
}
}
void RetracePath(Node startNode, Node endNode) {
List<Node> path = new List<Node>();
Node currentNode = endNode;
while (currentNode != startNode) {
path.Add(currentNode);
currentNode = currentNode.parent;
}
path.Reverse();
grid.path = path;
}
int GetDistance(Node nodeA, Node nodeB) {
int dstX = Mathf.Abs(nodeA.gridX - nodeB.gridX);
int dstY = Mathf.Abs(nodeA.gridY - nodeB.gridY);
if (dstX > dstY) {
return 14 * dstY + 10 * (dstX - dstY);
}
return 14 * dstX + 10 * (dstY - dstX);
}
}
```
该脚本中的A*寻路算法会在每次Update()函数调用时寻找从起点到终点的最短路径,并将其保存在网格的路径中。
实现A*寻路算法需要一个网格,该网格由一系列节点组成。每个节点包含了该节点在网格中的位置、该节点到起点的距离(gCost)、
阅读全文
相关推荐



















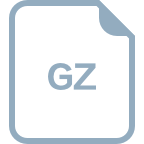