http post请求 jsonObject 工具类
时间: 2023-08-18 19:08:30 浏览: 107
以下是一个Java中发送HTTP POST请求并传递JSON对象的工具类:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import org.json.JSONObject;
public class HttpUtil {
public static String sendPost(String url, JSONObject json) throws Exception {
URL obj = new URL(url);
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
// 设置请求方法为POST
con.setRequestMethod("POST");
// 设置请求头部信息
con.setRequestProperty("Content-Type", "application/json");
con.setRequestProperty("Accept", "application/json");
// 允许输出流,即允许向服务器发送数据
con.setDoOutput(true);
// 创建JSON对象
JSONObject jsonObject = json;
// 将JSON对象转换成字符串
String jsonBody = jsonObject.toString();
// 获取输出流对象
OutputStream os = con.getOutputStream();
// 向输出流写入JSON字符串
os.write(jsonBody.getBytes());
// 刷新输出流,确保数据被发送到服务器
os.flush();
// 关闭输出流
os.close();
// 获取响应码
int responseCode = con.getResponseCode();
// 读取响应内容
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
// 关闭输入流
in.close();
// 返回响应结果
return response.toString();
}
}
```
使用时,只需调用`sendPost`方法即可:
```java
JSONObject json = new JSONObject();
json.put("key1", "value1");
json.put("key2", "value2");
String url = "http://example.com/api";
String response = HttpUtil.sendPost(url, json);
```
其中,`json`为要传递的JSON对象,`url`为要请求的URL地址,`response`为服务器返回的响应结果。
阅读全文
相关推荐
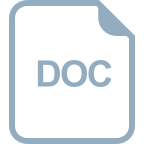
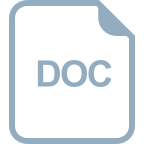
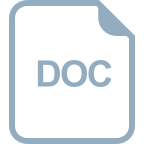
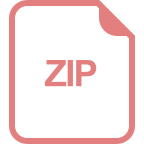
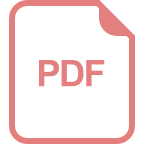
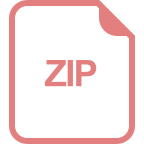
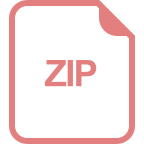
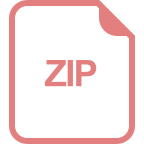
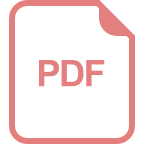
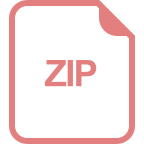
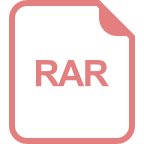
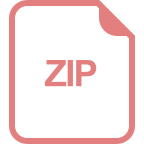
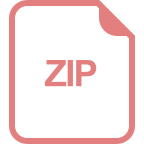
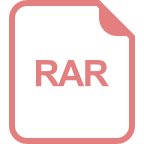
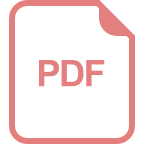
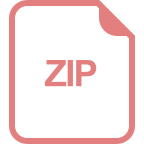
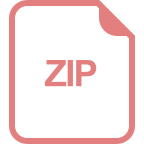
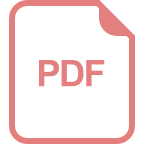
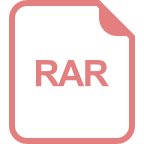