python实现将一张图片放大5倍,并实现将图片的设立阈值将红色部分二值化为1,其他部分为0,显示并保存
时间: 2023-06-13 07:02:09 浏览: 71
可以使用Python的Pillow库来实现图片的放大和二值化。
首先,需要安装Pillow库:
```python
pip install Pillow
```
然后,可以按照以下步骤实现:
1. 打开图片。
```python
from PIL import Image
img = Image.open('image.jpg')
```
2. 对图片进行放大。
```python
img = img.resize((img.width * 5, img.height * 5), Image.ANTIALIAS)
```
3. 将红色部分设为1,其他部分设为0。
```python
threshold = 128
img = img.convert('L').point(lambda x: int(x > threshold and img.getpixel((x, 0))[0] == 255), mode='1')
```
4. 显示并保存图片。
```python
img.show()
img.save('result.jpg')
```
完整代码如下:
```python
from PIL import Image
# 打开图片
img = Image.open('image.jpg')
# 放大图片
img = img.resize((img.width * 5, img.height * 5), Image.ANTIALIAS)
# 将红色部分设为1,其他部分设为0
threshold = 128
img = img.convert('L').point(lambda x: int(x > threshold and img.getpixel((x, 0))[0] == 255), mode='1')
# 显示并保存图片
img.show()
img.save('result.jpg')
```
阅读全文
相关推荐
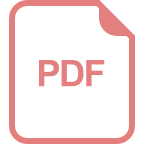

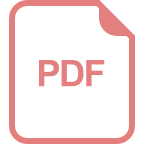
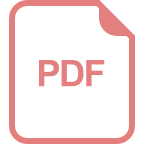
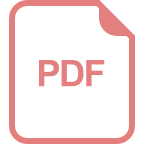
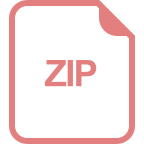
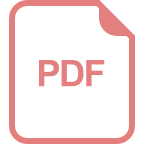
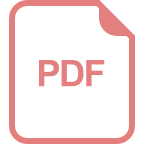
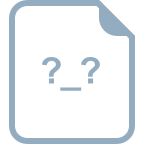
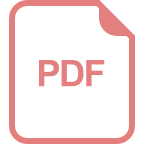
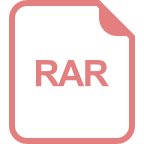
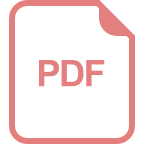
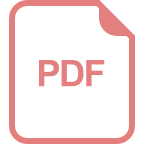
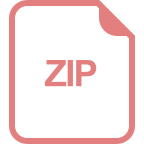
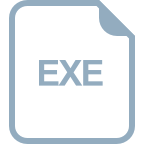
