C# 使用 HttpPost 请求调用 WebService
时间: 2024-04-21 13:23:57 浏览: 12
在 C# 中使用 HttpPost 请求调用 WebService,可以按照以下步骤进行操作:
1. 首先,确保你的 C# 项目中引用了 `System.Net.Http` 命名空间。
2. 创建一个 `HttpClient` 对象,用于发送 Http 请求。可以使用以下代码创建一个 `HttpClient` 对象:
```csharp
HttpClient client = new HttpClient();
```
3. 设置要调用的 WebService 的 URL。假设 WebService 的 URL 是 `http://example.com/your_webservice`,可以使用以下代码设置 URL:
```csharp
string url = "http://example.com/your_webservice";
```
4. 构建请求参数。根据你要调用的具体 WebService 方法的要求,构建相应的请求参数。可以使用 `FormUrlEncodedContent` 类来构建 URL 编码的请求参数。例如:
```csharp
var parameters = new Dictionary<string, string>
{
{ "param1", "value1" },
{ "param2", "value2" }
};
var content = new FormUrlEncodedContent(parameters);
```
5. 发送 HttpPost 请求并获取响应。使用 `client.PostAsync` 方法发送 HttpPost 请求,并使用 `await` 关键字等待响应。例如:
```csharp
HttpResponseMessage response = await client.PostAsync(url, content);
```
6. 处理响应。根据你的需求,可以从响应中获取内容、状态码等信息。例如,可以使用以下代码获取响应内容:
```csharp
string responseContent = await response.Content.ReadAsStringAsync();
```
这样,你就可以在 C# 中使用 HttpPost 请求调用 WebService。根据实际情况,可能还需要处理异常、设置请求头等操作。
相关推荐
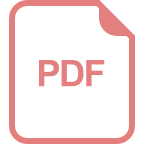











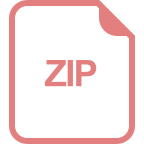
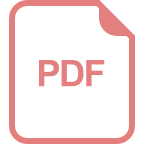
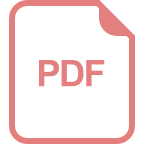
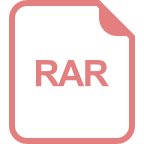