maxicode java
时间: 2024-05-12 21:21:13 浏览: 78
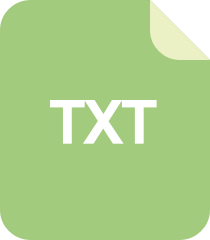
java code
Maxicode is a two-dimensional barcode that is used in the shipping industry to encode information such as recipient address, shipping method, and tracking number. In order to generate Maxicode in Java, you can use a library such as ZXing (Zebra Crossing).
Here's an example of how to generate a Maxicode using ZXing in Java:
```
import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.MultiFormatWriter;
import com.google.zxing.WriterException;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.maxicode.MaxiCodeWriter;
import java.util.HashMap;
public class MaxicodeExample {
public static void main(String[] args) {
String data = "123456789"; // the data to encode
int width = 300; // width of the barcode
int height = 300; // height of the barcode
// set up encoding hints
HashMap<EncodeHintType, Object> hints = new HashMap<>();
hints.put(EncodeHintType.MAX_SIZE, 300);
hints.put(EncodeHintType.MARGIN, 0);
// create Maxicode writer and encode the data
MaxiCodeWriter writer = new MaxiCodeWriter();
BitMatrix bitMatrix;
try {
bitMatrix = writer.encode(data, BarcodeFormat.MAXICODE, width, height, hints);
} catch (WriterException e) {
e.printStackTrace();
return;
}
// print the barcode as a string of asterisks
for (int y = 0; y < height; y++) {
StringBuilder row = new StringBuilder();
for (int x = 0; x < width; x++) {
if (bitMatrix.get(x, y)) {
row.append("*");
} else {
row.append(" ");
}
}
System.out.println(row);
}
}
}
```
This code creates a Maxicode barcode with the data "123456789" and a size of 300x300 pixels. It then prints out the barcode as a string of asterisks. You can modify the data and size as needed for your specific use case.
阅读全文
相关推荐
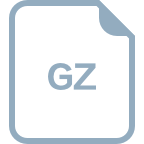
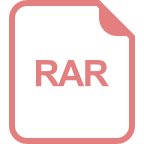
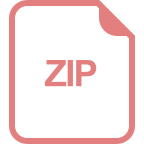
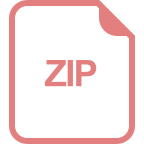
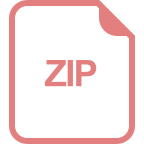

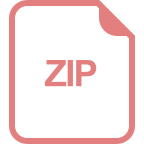
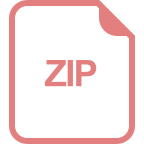
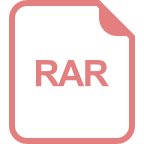
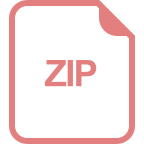