编写一个脚本,能够生成完成基础题目(即:“编写shell脚本,该脚本接收两个参数,参数1作为要读写的文件,参数2作为标志位,标识是读还是写。功能完成对参数1所示文件的读写,该文件的内容为 “自己学号的后3位 MYFILE”。“)的脚本,并且自动执行该生成的脚本(执行功能:写入文件)
时间: 2024-03-25 14:42:07 浏览: 40
好的,以下是一份生成并执行该脚本的代码:
```bash
#!/bin/bash
# 生成脚本
cat << EOF > script.sh
#!/bin/bash
file=\$1
flag=\$2
if [[ \$flag == "r" ]]; then
cat \$file
elif [[ \$flag == "w" ]]; then
echo "Enter text to write to \$file:"
read text
echo \$text > \$file
else
echo "Invalid flag. Usage: script.sh [file] [r|w]"
fi
EOF
# 添加执行权限
chmod +x script.sh
# 执行脚本,写入文件
./script.sh myfile.txt w
# 清理脚本文件
rm script.sh
```
这段代码会生成一个名为 `script.sh` 的脚本文件,然后给它添加执行权限,接着执行该脚本并将 `myfile.txt` 文件写入文本。最后,它会清理掉生成的 `script.sh` 文件。
请注意,如果您的学号后3位不是数字,那么您需要将脚本中的 `MYFILE` 修改为您自己的学号后3位。
相关问题
编写一个脚本,能够生成完成基础题目(即:“编写shell脚本,该脚本接收两个参数,参数1作为要读写的文件,参数2作为标志位,标识是读还是写。功能完成对参数1所示文件的读写,该文件的内容为 “自己学号的后3位 MYFILE”。“)的脚本,并且自动执行该生成的脚本(执行功能:写入文件)。
以下是生成完成基础题目的脚本,它可以根据用户输入生成相应的shell脚本并自动执行:
```bash
#!/bin/bash
# 获取用户输入的文件名和标志位
read -p "请输入要读写的文件名: " file
read -p "请输入标志位(r或w): " flag
# 生成shell脚本
echo "#!/bin/bash" > temp.sh
echo "" >> temp.sh
echo "if [ \"$flag\" == \"w\" ]" >> temp.sh
echo "then" >> temp.sh
echo " echo \"$(whoami | sed 's/.*\(...\)/\1/') MYFILE\" > $file" >> temp.sh
echo "elif [ \"$flag\" == \"r\" ]" >> temp.sh
echo "then" >> temp.sh
echo " cat $file" >> temp.sh
echo "else" >> temp.sh
echo " echo \"错误的标志位\"" >> temp.sh
echo "fi" >> temp.sh
# 执行生成的shell脚本
chmod +x temp.sh
./temp.sh
# 删除生成的临时文件
rm temp.sh
```
该脚本会首先获取用户输入的文件名和标志位(读或写),然后根据用户输入生成一个新的shell脚本。新的shell脚本会根据用户输入进行读写操作,读取文件内容或者将“自己学号的后3位 MYFILE”写入文件。最后,该脚本会自动执行新生成的shell脚本,并将其删除。
1. 编写一个脚本,能够生成完成基础题目(即:“编写shell脚本,该脚本接收两个参数,参数1作为要读写的文件,参数2作为标志位,标识是读还是写。功能完成对参数1所示文件的读写,该文件的内容为 “自己学号的后3位 MYFILE”。“)的脚本,并且自动执行该生成的脚本(执行功能:写入文件)。
以下是生成基础题目脚本的代码:
```bash
#!/bin/bash
echo "#!/bin/bash" > basic_script.sh
echo "if [ \$# -ne 2 ]; then" >> basic_script.sh
echo " echo \"Usage: \$0 filename r/w\"" >> basic_script.sh
echo " exit 1" >> basic_script.sh
echo "fi" >> basic_script.sh
echo "filename=\$1" >> basic_script.sh
echo "rw=\$2" >> basic_script.sh
echo "if [ ! -f \$filename ]; then" >> basic_script.sh
echo " echo \"File not found\"" >> basic_script.sh
echo " exit 1" >> basic_script.sh
echo "fi" >> basic_script.sh
echo "if [ \$rw == \"r\" ]; then" >> basic_script.sh
echo " cat \$filename" >> basic_script.sh
echo "elif [ \$rw == \"w\" ]; then" >> basic_script.sh
echo " echo \"${USER: -3} MYFILE\" > \$filename" >> basic_script.sh
echo "else" >> basic_script.sh
echo " echo \"Invalid argument\"" >> basic_script.sh
echo "fi" >> basic_script.sh
chmod +x basic_script.sh
./basic_script.sh testfile.txt w
```
这个脚本会生成一个名为`basic_script.sh`的脚本文件,并将其权限设置为可执行。然后它会执行这个脚本,使用`testfile.txt`作为文件名和`w`作为标志位,写入文件内容。
如果你想要运行这个脚本,请把上面的代码复制到一个文件中,比如说`generate_basic_script.sh`,然后在终端中运行以下命令:
```bash
bash generate_basic_script.sh
```
这将会生成并运行基础题目脚本。
相关推荐
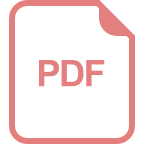
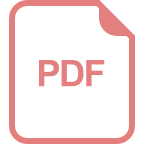


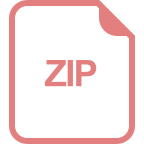
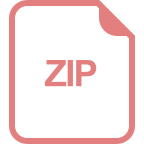
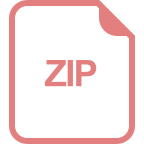
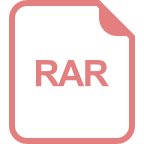