java实现远程导出导入mysql文件
时间: 2024-09-23 20:07:32 浏览: 32
在Java中,要实现远程导出和导入MySQL数据库文件,你可以使用JDBC (Java Database Connectivity) 来连接数据库,然后配合一些第三方库如`com.mysql.jdbc`或`mysql-connector-java`来进行文件操作。以下是基本步骤:
**远程导出MySQL数据到CSV文件:**
```java
import java.io.FileWriter;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
public void exportToCsv(String url, String user, String password, String dbName, String tableName, String outputPath) {
try {
Class.forName("com.mysql.cj.jdbc.Driver");
Connection conn = DriverManager.getConnection(url, user, password);
Statement stmt = conn.createStatement();
// 执行SQL查询获取所有数据
ResultSet rs = stmt.executeQuery("SELECT * FROM " + dbName + "." + tableName);
FileWriter writer = new FileWriter(outputPath);
while (rs.next()) {
for (int i = 0; i < rs.getMetaData().getColumnCount(); i++) {
writer.append(rs.getString(i+1)).append(",");
}
writer.append("\n");
}
rs.close();
stmt.close();
conn.close();
System.out.println("Export completed to " + outputPath);
} catch (Exception e) {
e.printStackTrace();
}
}
```
**远程导入CSV文件到MySQL数据库:**
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.sql.Connection;
import java.sql.PreparedStatement;
public void importFromCsv(String url, String user, String password, String dbName, String tableName, String inputFile) {
try {
Class.forName("com.mysql.cj.jdbc.Driver");
Connection conn = DriverManager.getConnection(url, user, password);
PreparedStatement pstmt = conn.prepareStatement(
"INSERT INTO " + dbName + "." + tableName + " VALUES (?, ?, ?)"
);
BufferedReader reader = new BufferedReader(new FileReader(inputFile));
String line;
while ((line = reader.readLine()) != null) {
String[] values = line.split(",");
pstmt.setString(1, values[0]);
pstmt.setString(2, values[1]); // 假设表的第一列和第二列为字符串字段
pstmt.setInt(3, Integer.parseInt(values[2])); // 如果第三列为数字类型
pstmt.addBatch();
}
pstmt.executeBatch();
reader.close();
pstmt.close();
conn.commit();
conn.close();
System.out.println("Import completed from " + inputFile);
} catch (Exception e) {
e.printStackTrace();
}
}
```
相关推荐
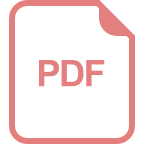
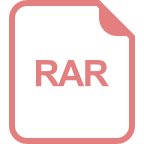
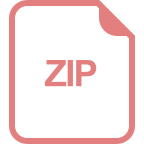















