js数组查找元素 findindex() 方法,返回第一个匹配元素下标
时间: 2023-05-04 13:02:49 浏览: 85
JavaScript 中的数组查找方法 findIndex() 可以在数组中查找匹配元素并返回其下标。这个方法接收一个回调函数作为参数,用于指定查找的方式。
回调函数接收三个参数:数组元素,元素下标和数组本身。当查找到第一个匹配元素时,findIndex() 方法会返回该元素的下标,如果没有找到则返回 -1。
例如,我们可以使用 findIndex() 方法来查找数组中第一个大于等于 5 的元素下标:
```
const array = [1, 3, 4, 5, 6, 7];
const index = array.findIndex((element) => element >= 5);
console.log(index); // 输出 3
```
在这个例子中,我们给 findIndex() 方法传入了一个回调函数,回调函数判断数组元素是否大于等于 5。因为数组中第一个大于等于 5 的元素下标是 3,所以 findIndex() 方法返回了 3。
需要注意的是,findIndex() 方法只会返回第一个匹配元素的下标,如果需要查找出所有匹配的元素下标,可以使用 filter() 方法配合使用。
相关问题
js代码-js数组查找元素 findindex() 方法,返回第一个匹配元素下标
JavaScript中,数组是一种常见的数据类型。当我们需要查找数组中特定元素的索引位置时,可以使用数组的findIndex()方法。这个方法会查找数组中满足条件的第一个元素,并返回它的索引值。
findIndex()方法接收一个回调函数作为参数,这个回调函数的作用是对数组中的每个元素进行判断,返回布尔值。如果返回true,那么这个元素的索引值就会作为findIndex()方法的返回值。
例如,我们想要在以下数组中查找第一个大于10的元素的索引位置:
var arr = [5, 8, 11, 13, 9];
我们可以使用以下代码实现:
var index = arr.findIndex(function(item) {
return item > 10;
});
console.log(index); // 2
这个代码中,回调函数判断数组中的每个元素,如果元素的值大于10,则返回true,这个元素的索引值就会作为findIndex()方法的返回值。因为11是第一个大于10的元素,所以最终返回的值是2,代表该元素在数组中的位置。
除了findIndex()方法,还有许多其它的数组查找方法可以使用,例如find()方法、indexOf()方法等等。在编写JavaScript代码时,我们可以根据实际需要选择合适的方法来完成数组查找的任务。
js代码-js数组查找元素 find() 方法,只返回第一个匹配元素
JS数组查找元素是开发中经常使用的一种操作,而find()方法是其中比较常用的一种方式。这个方法通过遍历数组找出符合条件的第一个元素,但是只会返回第一个匹配元素,不会返回其他匹配元素。因此,在使用find()方法时需要注意这一点。
find()方法在使用时,需要提供一个回调函数作为参数。这个回调函数会接收三个参数:数组元素,元素索引和数组本身。回调函数必须返回true或者false来指示当前元素是否匹配要求。
当find()方法找到第一个匹配元素时,就会停止遍历整个数组,因此即使数组中存在多个匹配元素,也只会返回第一个匹配元素。
在实际开发中,如果需要查找所有匹配元素,可以使用filter()方法来实现。filter()方法返回一个包含所有匹配元素的新数组。
综上所述,当使用find()方法查找元素时,需要注意该方法只会返回第一个匹配元素,如果需要找到所有匹配元素,可以使用filter()方法。
相关推荐
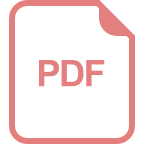
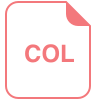
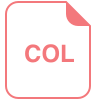
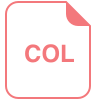
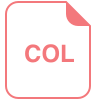







