写一个运行于arm linux系统的c++代码片段,判断目录/mnt/log是否存在,如果不存在就创建它。要求不使用std::filesystem库
时间: 2024-12-19 19:14:49 浏览: 37
在ARM Linux系统上,你可以使用`<unistd.h>`和`<sys/stat.h>`头文件来进行目录的路径操作,包括检查其存在性和创建新目录。下面是一个简单的C++代码片段:
```cpp
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#include <errno.h>
// 定义路径
const char* dir_path = "/mnt/log";
int main() {
struct stat sb;
int ret;
// 检查目录是否存在
ret = lstat(dir_path, &sb);
if (ret == -1) {
if (errno == ENOENT) { // 如果错误码表示路径不存在
printf("Directory %s does not exist.\n", dir_path);
// 创建目录
mode_t mode = S_IRWXU | S_IRGRP | S_IXGRP | S_IROTH | S_IXOTH; // 设置权限
ret = mkdir(dir_path, mode);
if (ret != 0) {
perror("mkdir failed");
return 1;
}
printf("Directory %s created successfully.\n", dir_path);
} else {
perror("lstat failed");
return 1;
}
} else {
printf("Directory %s exists.\n", dir_path);
}
return 0;
}
```
这个程序首先尝试获取指定目录的信息,如果返回失败且错误码是`ENOENT`,则说明目录不存在并尝试创建。如果创建成功,会打印相关信息;如果创建失败,则输出错误信息。
阅读全文
相关推荐




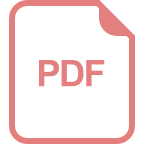







