如何把yolov7检测视频后生成图像保存
时间: 2023-11-25 11:05:56 浏览: 403
要将 YOLOv7 检测视频后生成的图像保存下来,可以按照以下步骤进行操作:
1. 使用 YOLOv7 模型对视频进行检测,得到检测结果。
2. 遍历检测结果,将每个检测框对应的图像区域保存下来。
3. 将保存下来的图像使用 Python 中的 OpenCV 库进行保存。
以下是一个简单的示例代码,可以将 YOLOv7 检测视频后生成的图像保存到本地:
``` python
import cv2
import numpy as np
# 加载 YOLOv7 模型
net = cv2.dnn.readNetFromDarknet('yolov7.cfg', 'yolov7.weights')
# 加载类别标签
classes = []
with open('coco.names', 'r') as f:
classes = [line.strip() for line in f.readlines()]
# 加载视频并设置输出视频格式
cap = cv2.VideoCapture('input.mp4')
fourcc = cv2.VideoWriter_fourcc(*'XVID')
out = cv2.VideoWriter('output.avi', fourcc, 30.0, (int(cap.get(3)), int(cap.get(4))))
# 循环读取视频帧
while True:
# 读取视频帧
ret, frame = cap.read()
if not ret:
break
# 对视频帧进行检测
blob = cv2.dnn.blobFromImage(frame, 1/255.0, (416, 416), swapRB=True, crop=False)
net.setInput(blob)
outs = net.forward(net.getUnconnectedOutLayersNames())
# 处理检测结果
class_ids = []
confidences = []
boxes = []
for out in outs:
for detection in out:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5:
center_x = int(detection[0] * frame.shape[1])
center_y = int(detection[1] * frame.shape[0])
width = int(detection[2] * frame.shape[1])
height = int(detection[3] * frame.shape[0])
left = int(center_x - width / 2)
top = int(center_y - height / 2)
class_ids.append(class_id)
confidences.append(float(confidence))
boxes.append([left, top, width, height])
# 遍历检测结果,保存每个检测框对应的图像区域
for i in range(len(boxes)):
left, top, width, height = boxes[i]
roi = frame[top:top+height, left:left+width]
cv2.imwrite('output_{}.jpg'.format(i), roi)
# 将处理后的视频帧写入输出视频
out.write(frame)
# 释放资源
cap.release()
out.release()
cv2.destroyAllWindows()
```
上面的代码将视频帧逐一检测,并将每个检测框对应的图像区域保存为单独的图像文件,文件名为 `output_0.jpg`、`output_1.jpg` 等。如果要将所有图像保存到一个文件夹中,可以将保存路径修改为某个文件夹即可。
阅读全文
相关推荐
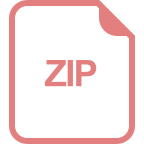
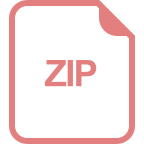



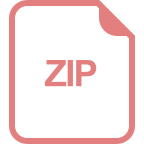
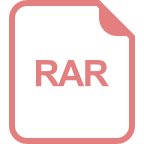
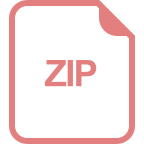
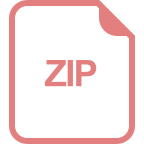
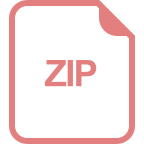
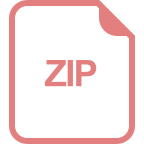
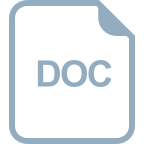
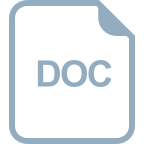
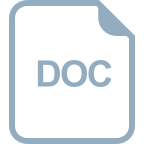
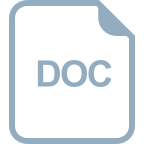

