函数的静态绑定和动态绑定,举例说明
时间: 2024-02-19 22:03:16 浏览: 79
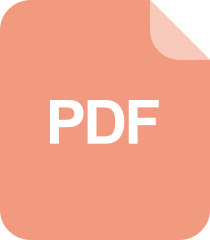
java动态绑定和静态绑定用法实例详解

好的,我再举两个更具体的例子:
1. 静态绑定的例子:
```c++
#include <iostream>
class Animal {
public:
void makeSound() {
std::cout << "Animal makes a sound" << std::endl;
}
};
class Cat : public Animal {
public:
void makeSound() {
std::cout << "Cat meows" << std::endl;
}
};
int main() {
Animal* animal = new Cat();
animal->makeSound(); // 输出 Animal makes a sound
delete animal;
return 0;
}
```
这个例子中,Animal和Cat都是类,Cat继承自Animal。在main函数中,我们创建了一个Animal指针指向Cat对象,然后调用它的makeSound方法。由于makeSound方法是虚函数,因此会根据运行时的实际对象类型来调用相应的方法。但是,由于这里使用的是静态绑定,编译器在编译的时候已经决定了调用的方法是Animal的makeSound方法,因此输出的是"Animal makes a sound",而不是"Cat meows"。
2. 动态绑定的例子:
```c++
#include <iostream>
class Animal {
public:
virtual void makeSound() {
std::cout << "Animal makes a sound" << std::endl;
}
};
class Cat : public Animal {
public:
void makeSound() override {
std::cout << "Cat meows" << std::endl;
}
};
int main() {
Animal* animal = new Cat();
animal->makeSound(); // 输出 Cat meows
delete animal;
return 0;
}
```
这个例子与上面的例子非常类似,唯一的区别在于Animal类中的makeSound方法被声明为了虚函数,并使用了override关键字。这样一来,在运行时期,程序就可以根据实际对象类型来决定调用哪个方法,这就是动态绑定。因此,输出的结果就是"Cat meows"。
阅读全文
相关推荐
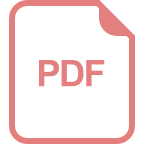
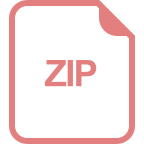





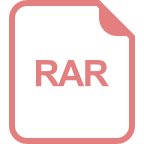
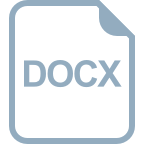
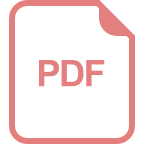
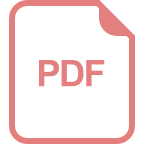
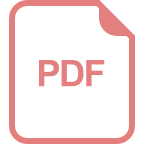
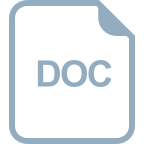
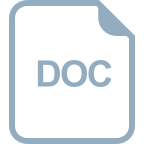


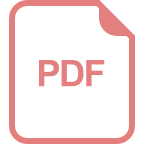