使用JAVA写一段代码,要求写出衣服管理系统
时间: 2023-12-29 11:02:23 浏览: 153
好的,以下是一个简单的衣服管理系统的Java代码:
```java
import java.util.Scanner;
public class ClothesManagementSystem {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int option = 0;
int num = 0;
Clothes[] clothes = new Clothes[100]; // 最多可以管理100件衣服
while (true) {
System.out.println("欢迎使用衣服管理系统,请选择以下操作:");
System.out.println("1. 添加衣服");
System.out.println("2. 删除衣服");
System.out.println("3. 修改衣服信息");
System.out.println("4. 查询衣服");
System.out.println("5. 退出系统");
System.out.print("请选择操作:");
option = input.nextInt();
switch (option) {
case 1:
System.out.print("请输入衣服名字:");
String name = input.next();
System.out.print("请输入衣服价格:");
double price = input.nextDouble();
System.out.print("请输入衣服颜色:");
String color = input.next();
System.out.print("请输入衣服尺寸:");
String size = input.next();
Clothes newClothes = new Clothes(name, price, color, size);
clothes[num++] = newClothes;
System.out.println("添加成功!");
break;
case 2:
System.out.print("请输入要删除的衣服名字:");
String delName = input.next();
boolean isDeleted = false;
for (int i = 0; i < num; i++) {
if (clothes[i].getName().equals(delName)) {
for (int j = i; j < num - 1; j++) {
clothes[j] = clothes[j + 1];
}
num--;
isDeleted = true;
break;
}
}
if (isDeleted) {
System.out.println("删除成功!");
} else {
System.out.println("没有找到该衣服!");
}
break;
case 3:
System.out.print("请输入要修改的衣服名字:");
String modifyName = input.next();
boolean isModified = false;
for (int i = 0; i < num; i++) {
if (clothes[i].getName().equals(modifyName)) {
System.out.print("请输入衣服价格:");
clothes[i].setPrice(input.nextDouble());
System.out.print("请输入衣服颜色:");
clothes[i].setColor(input.next());
System.out.print("请输入衣服尺寸:");
clothes[i].setSize(input.next());
isModified = true;
break;
}
}
if (isModified) {
System.out.println("修改成功!");
} else {
System.out.println("没有找到该衣服!");
}
break;
case 4:
System.out.print("请输入要查询的衣服名字:");
String queryName = input.next();
boolean isFound = false;
for (int i = 0; i < num; i++) {
if (clothes[i].getName().equals(queryName)) {
System.out.println(clothes[i]);
isFound = true;
break;
}
}
if (!isFound) {
System.out.println("没有找到该衣服!");
}
break;
case 5:
System.out.println("谢谢使用衣服管理系统!");
System.exit(0);
default:
System.out.println("输入有误,请重新选择!");
break;
}
}
}
}
class Clothes {
private String name;
private double price;
private String color;
private String size;
public Clothes(String name, double price, String color, String size) {
this.name = name;
this.price = price;
this.color = color;
this.size = size;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public String getSize() {
return size;
}
public void setSize(String size) {
this.size = size;
}
@Override
public String toString() {
return "衣服名字:" + name + ",价格:" + price + ",颜色:" + color + ",尺寸:" + size;
}
}
```
这个衣服管理系统可以实现添加、删除、修改和查询衣服的功能,使用了基本的面向对象编程思想。用户可以根据自己的需要对衣服进行操作。
阅读全文
相关推荐
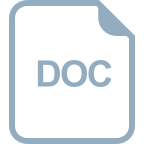
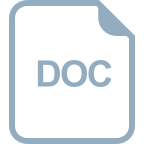
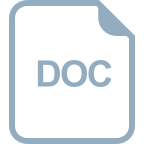
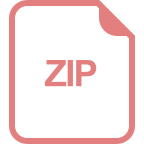
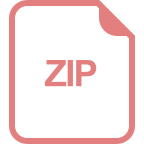
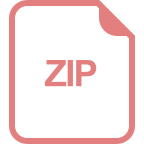
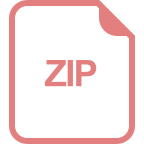
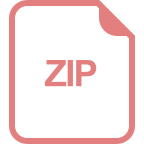
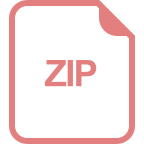
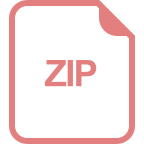
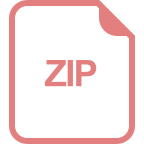
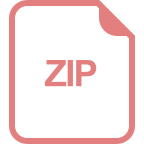
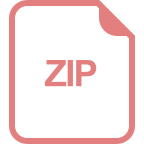
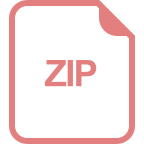
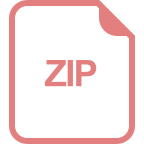
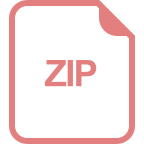