LRU算法python代码
时间: 2023-03-30 11:02:21 浏览: 150
以下是 LRU 算法的 Python 代码:
```python
class LRUCache:
def __init__(self, capacity: int):
self.capacity = capacity
self.cache = {}
self.order = []
def get(self, key: int) -> int:
if key in self.cache:
self.order.remove(key)
self.order.append(key)
return self.cache[key]
else:
return -1
def put(self, key: int, value: int) -> None:
if key in self.cache:
self.order.remove(key)
elif len(self.cache) == self.capacity:
del self.cache[self.order.pop()]
self.cache[key] = value
self.order.append(key)
```
希望对你有帮助!
相关问题
pythonLRU算法
Python LRU算法是一种缓存淘汰策略,它基于最近最少使用原则,即当缓存满时,会优先淘汰最近最少使用的缓存。
下面是一个简单的Python LRU算法实现:
```python
from collections import OrderedDict
class LRUCache:
def __init__(self, capacity: int):
self.cache = OrderedDict()
self.capacity = capacity
def get(self, key: int) -> int:
if key not in self.cache:
return -1
self.cache.move_to_end(key)
return self.cache[key]
def put(self, key: int, value: int) -> None:
if key in self.cache:
self.cache.move_to_end(key)
self.cache[key] = value
if len(self.cache) > self.capacity:
self.cache.popitem(last=False)
```
在上述实现中,我们使用了Python中的OrderedDict类来实现LRU算法。该类内部维护了一个有序字典,其中最近访问的元素会被移动到字典的末尾,而最久未访问的元素会被移动到字典的开头。当缓存已满时,我们可以通过popitem()方法将字典开头的元素删除,从而实现缓存淘汰的目的。在get()和put()方法中,我们都会将访问的元素移动到字典的末尾,以保证最近访问的元素永远在字典的末尾。
lru页面置换算法python
LRU (Least Recently Used) 页面置换算法是一种常用的计算机内存管理策略,主要用于解决虚拟内存中的缓存替换问题。当系统内存不足时,它会淘汰最近最少使用的页面,以便腾出空间给新访问的数据。
在Python中,虽然标准库并未直接提供对LRU算法的实现,但我们可以通过第三方库如`cachetools`来轻松地应用这个概念。`cachetools.lru`模块提供了LRU Cache的功能,例如:
```python
from cachetools import LRUCache
# 创建一个大小为10的LRU缓存
cache = LRUCache(maxsize=10)
# 存储数据
cache['key1'] = 'value1'
cache['key2'] = 'value2'
# 如果缓存已满,最近最少使用的键会被自动移除,比如 'key3' 被替换掉
cache['key3'] = 'value3'
# 获取值
print(cache.get('key1')) # 输出 'value1'
```
当你尝试获取一个不存在于缓存中的键时,`get()`函数会返回None。这就是LRU缓存的工作原理。
阅读全文
相关推荐
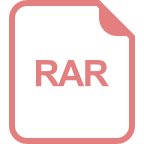
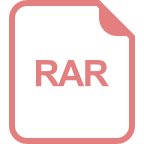
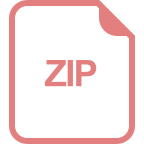
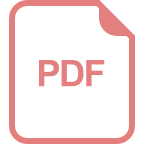
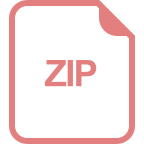
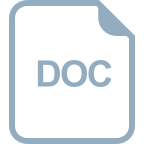
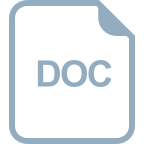
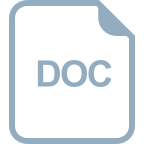






