帮我写一段用yolo进行回归预测的代码
时间: 2023-06-16 10:02:40 浏览: 50
抱歉,YOLO算法用于目标检测而不是回归预测。如果您需要使用YOLO进行目标检测,可以使用以下代码:
```python
import cv2
import numpy as np
import argparse
# 构建参数解析器
ap = argparse.ArgumentParser()
ap.add_argument("-i", "--image", required=True, help="path to input image")
ap.add_argument("-y", "--yolo", required=True, help="base path to YOLO directory")
ap.add_argument("-c", "--confidence", type=float, default=0.5, help="minimum probability to filter weak detections")
ap.add_argument("-t", "--threshold", type=float, default=0.3, help="threshold when applying non-maxima suppression")
args = vars(ap.parse_args())
# 加载 YOLO 模型
weights_path = os.path.sep.join([args["yolo"], "yolov3.weights"])
config_path = os.path.sep.join([args["yolo"], "yolov3.cfg"])
net = cv2.dnn.readNetFromDarknet(config_path, weights_path)
# 加载图像,获取图像尺寸,并构建一个 blob 用于输入网络
image = cv2.imread(args["image"])
(H, W) = image.shape[:2]
blob = cv2.dnn.blobFromImage(image, 1 / 255.0, (416, 416), swapRB=True, crop=False)
# 设置网络输入和输出层名称
net.setInput(blob)
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
# 前向传递,获取边界框和置信度
outputs = net.forward(output_layers)
boxes = []
confidences = []
class_ids = []
for output in outputs:
for detection in output:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > args["confidence"]:
box = detection[0:4] * np.array([W, H, W, H])
(centerX, centerY, width, height) = box.astype("int")
x = int(centerX - (width / 2))
y = int(centerY - (height / 2))
boxes.append([x, y, int(width), int(height)])
confidences.append(float(confidence))
class_ids.append(class_id)
# 应用非最大值抑制
idxs = cv2.dnn.NMSBoxes(boxes, confidences, args["confidence"], args["threshold"])
# 绘制边界框和标签
if len(idxs) > 0:
for i in idxs.flatten():
(x, y) = (boxes[i][0], boxes[i][1])
(w, h) = (boxes[i][2], boxes[i][3])
cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
text = "{}: {:.4f}".format(classes[class_ids[i]], confidences[i])
cv2.putText(image, text, (x, y - 5), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 显示结果
cv2.imshow("Image", image)
cv2.waitKey(0)
```
这个代码可以使用YOLOv3算法来检测输入图像中的物体,并在图像上绘制边界框和标签。注意,这段代码需要使用预先训练好的权重文件和配置文件,您需要指定这些文件的路径。
相关推荐
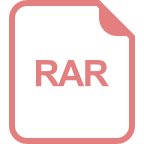
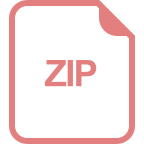
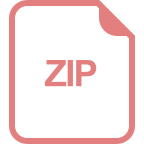










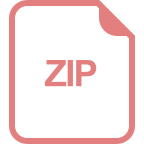
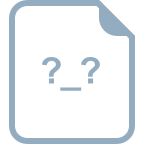