tensorflow并行多分支结构?
时间: 2023-07-30 07:04:49 浏览: 47
在 TensorFlow 中实现并行多分支结构可以通过使用 TensorFlow 的控制流操作和并行化技术来实现。以下是一种常见的方法:
1. 使用 `tf.cond()` 函数来实现条件控制流。该函数接受一个布尔条件和两个函数作为参数,并根据条件选择执行其中一个函数。你可以使用这个函数来创建多个分支,并在每个分支中执行不同的操作。
```python
import tensorflow as tf
def branch_1():
# 执行第一个分支操作的代码
...
def branch_2():
# 执行第二个分支操作的代码
...
condition = tf.placeholder(dtype=tf.bool)
output = tf.cond(condition, branch_1, branch_2)
```
在上面的代码中,`condition` 是一个布尔占位符,你可以在运行时将其设置为 `True` 或 `False` 来选择执行哪个分支。
2. 使用 `tf.while_loop()` 函数来实现循环控制流。该函数接受一个循环条件和一个函数作为参数,并重复执行该函数直到循环条件为假。你可以在函数内部实现多个分支,并使用 `tf.cond()` 来根据条件选择执行不同的操作。
```python
import tensorflow as tf
def loop_body(i, total):
# 执行循环体操作的代码
...
# 更新循环变量 i 和循环条件 total
i = tf.add(i, 1)
total = tf.add(total, 1)
return i, total
def loop_condition(i, total):
# 定义循环条件,例如 i < 10
return tf.less(i, 10)
initial_i = tf.constant(0)
initial_total = tf.constant(0)
final_i, final_total = tf.while_loop(loop_condition, loop_body, [initial_i, initial_total])
```
在上面的代码中,`loop_condition` 函数定义了循环的条件,`loop_body` 函数定义了循环体的操作。通过不断更新循环变量 `i` 和循环条件 `total`,可以实现多个分支的并行化。
这些方法可以帮助你在 TensorFlow 中实现并行多分支结构。根据你的具体需求,你可以根据这些方法进行适当的修改和扩展。
相关推荐
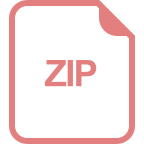
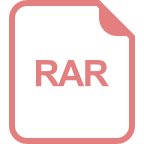
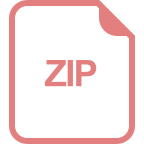














