python 日期格式去除时分秒
时间: 2023-07-25 08:35:04 浏览: 424
在 Python 中,可以使用 datetime 模块来处理日期时间。如果要从日期时间中去除时分秒,可以使用以下方法:
```python
from datetime import datetime
# 获取当前日期时间
now = datetime.now()
# 去除时分秒
date_only = now.date()
# 输出日期
print(date_only)
```
输出结果为:
```
2021-10-14
```
如果你已经有一个日期时间字符串,可以先将其解析为 datetime 对象,然后再去除时分秒。例如:
```python
from datetime import datetime
# 日期时间字符串
date_str = "2021-10-14 15:30:45"
# 解析为 datetime 对象
date_time = datetime.strptime(date_str, "%Y-%m-%d %H:%M:%S")
# 去除时分秒
date_only = date_time.date()
# 输出日期
print(date_only)
```
输出结果为:
```
2021-10-14
```
其中,`strptime` 方法用于将日期时间字符串解析为 datetime 对象,`"%Y-%m-%d %H:%M:%S"` 是表示日期时间格式的字符串。
相关问题
SQL中如何对字段中的时间去除时分秒
在SQL中,如果你想从日期时间字段中去除时分秒部分,通常可以使用`DATE_TRUNC`函数或者类似的操作。这个操作取决于你使用的数据库系统,以下是几种常见数据库系统的处理方式:
1. **MySQL** 和 **PostgreSQL**:
```
SELECT DATE_FORMAT(your_date_column, '%Y-%m-%d') AS date_without_time
FROM your_table;
```
2. **SQL Server**:
```
SELECT DATEPART(year, your_date_column) + '-' +
RIGHT(STR(DATEPART(month, your_date_column), 2), 2) + '-' +
RIGHT(STR(DATEPART(day, your_date_column), 2), 2) AS date_without_time
FROM your_table;
```
3. **Oracle**:
```
SELECT TRUNC(your_date_column, 'DAY') AS date_without_time
FROM your_table;
```
4. **SQLite** (如果可用的话,因为SQLite的日期函数有限,你可能需要借助其他语言如Python来处理):
```python
import sqlite3
conn = sqlite3.connect('your_database.db')
cursor = conn.cursor()
cursor.execute("SELECT datetime(your_date_column, 'unixepoch', 'localtime') - interval '1 second' as date_without_time FROM your_table")
result = cursor.fetchall()
```
记得将`your_date_column`替换为实际的字段名,`your_table`替换为表名。
对<text class="time-ui">活动时间:{{item.start_date}}--{{item.end_date}}</text>中的item.start_date去除时分秒
可以使用Python的datetime模块来处理日期和时间,具体操作如下:
```python
from datetime import datetime
start_date = datetime.strptime(item.start_date, '%Y-%m-%d %H:%M:%S')
start_date = start_date.date()
end_date = datetime.strptime(item.end_date, '%Y-%m-%d %H:%M:%S')
end_date = end_date.date()
# 格式化输出日期
print('活动时间:{}--{}'.format(start_date.strftime('%Y-%m-%d'), end_date.strftime('%Y-%m-%d')))
```
上述代码将item.start_date和item.end_date转换为datetime对象,然后使用date()方法获取日期部分,并使用strftime()方法将日期格式化为'%Y-%m-%d'的字符串,最后输出格式化后的活动时间。
阅读全文
相关推荐
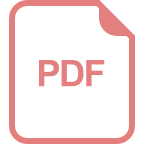
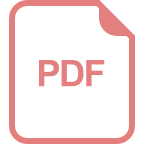
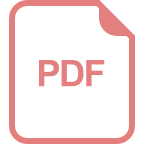
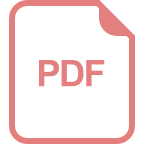
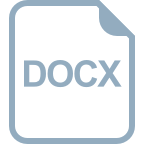
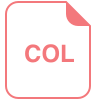
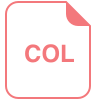


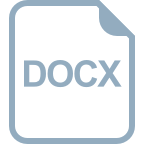
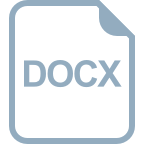
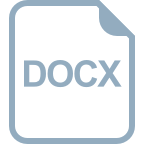